Python: Find smallest and largest word in a given string
Find smallest and largest words.
Write a Python program to find the smallest and largest words in a given string.
Vasual Presentation:
Flowchart:
Sample Solution:
Python Code:
# Function to find smallest and largest word
def smallest_largest_words(str1):
word = ""
all_words = [];
# Add space to end to capture last word
str1 = str1 + " "
# Split to words
for i in range(0, len(str1)):
if(str1[i] != ' '):
word = word + str1[i];
else:
all_words.append(word);
word = "";
# Initialize small and large
small = large = all_words[0];
# Find smallest and largest
for k in range(0, len(all_words)):
if(len(small) > len(all_words[k])):
small = all_words[k];
if(len(large) < len(all_words[k])):
large = all_words[k];
return small,large;
# Test string
str1 = "Write a Java program to sort an array of given integers using Quick sort Algorithm.";
# Print original string
print("Original Strings:\n",str1)
# Find smallest and largest words
small, large = smallest_largest_words(str1)
# Print result
print("Smallest word: " + small);
print("Largest word: " + large);
Sample Output:
Original Strings: Write a Java program to sort an array of given integers using Quick sort Algorithm. Smallest word: a Largest word: Algorithm.
Flowchart:
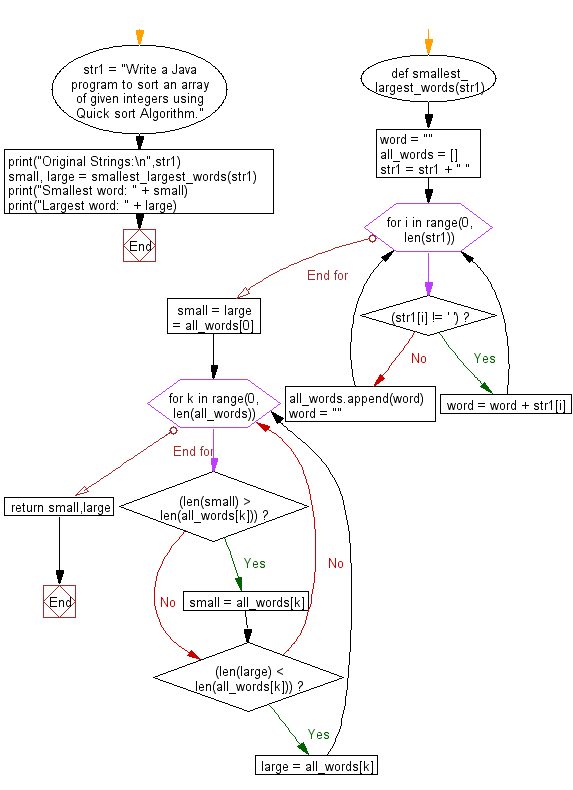
For more Practice: Solve these Related Problems:
- Write a Python program to identify the shortest and longest words in a sentence using split() and min()/max() with a custom key.
- Write a Python program to iterate through a list of words and return the word with the minimum and maximum length.
- Write a Python program to use sorted() with a key based on word length and return the first and last elements as the smallest and largest words.
- Write a Python program to implement a function that processes a string and outputs both the smallest and largest words in a case-insensitive manner.
Python Code Editor:
Previous: Write a Python program to count characters at same position in a given string (lower and uppercase characters) as in English alphabet.
Next: Write a Python program to count number of substrings with same first and last characters of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics