Python: Create two strings from a given string
Separate single and multiple occurrence chars.
Write a Python program to generate two strings from a given string. For the first string, use the characters that occur only once, and for the second, use the characters that occur multiple times in the said string.
Visual Presentation:
Sample Solution:
Python Code:
# Import Counter from collections
from collections import Counter
# Function to generate two strings
def generateStrings(input):
# Create a character counter from input
str_char_ctr = Counter(input)
# Part 1 contains single occurrence characters
part1 = [key for (key,count) in str_char_ctr.items() if count==1]
# Part 2 contains multiple occurrence characters
part2 = [key for (key,count) in str_char_ctr.items() if count>1]
# Sort the characters in each part
part1.sort()
part2.sort()
return part1,part2
# Test input string
input = "aabbcceffgh"
# Generate the two strings
s1, s2 = generateStrings(input)
# Print first string
print(''.join(s1))
# Print second string
print(''.join(s2))
Sample Output:
egh abcf
Flowchart:
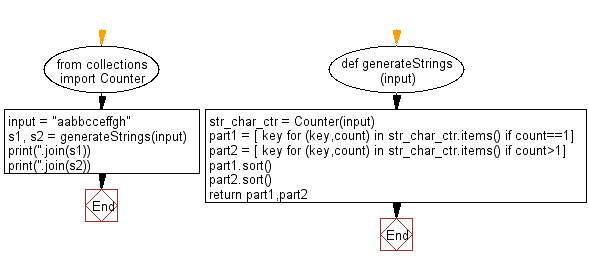
For more Practice: Solve these Related Problems:
- Write a Python program to partition a string into two strings: one containing characters that occur only once and another with characters that occur more than once.
- Write a Python program to iterate over a string and build two lists, one for unique characters and one for duplicates, then return them as strings.
- Write a Python program to use collections.Counter to separate characters into single-occurrence and multiple-occurrence groups.
- Write a Python program to filter the characters of a string into two distinct strings based on their frequency counts.
Go to:
Previous: Write a Python program to remove all consecutive duplicates of a given string.
Next: Write a Python program to find the longest common sub-string from two given strings.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.