Python: Remove all consecutive duplicates of a given string
Remove consecutive duplicates in string.
Write a Python program to remove all consecutive duplicates of a given string.
Visual Presentation:
Sample Solution - 1:
Python Code:
# Import groupby from itertools
from itertools import groupby
# Function to remove consecutive duplicates
def remove_all_consecutive(str1):
# Initialize empty result string
result_str = []
# Group string into consecutive characters
for (key,group) in groupby(str1):
# Append only one instance of each character
result_str.append(key)
# Join characters into string
return ''.join(result_str)
# Test string
str1 = 'xxxxxyyyyy'
# Print original string
print("Original string:" + str1)
# Print string to modify
print("After removing consecutive duplicates:")
# Print modified string
print(remove_all_consecutive(str1))
Sample Output:
Original string:xxxxxyyyyy After removing consecutive duplicates: xxxxxyyyyy xy
Flowchart:
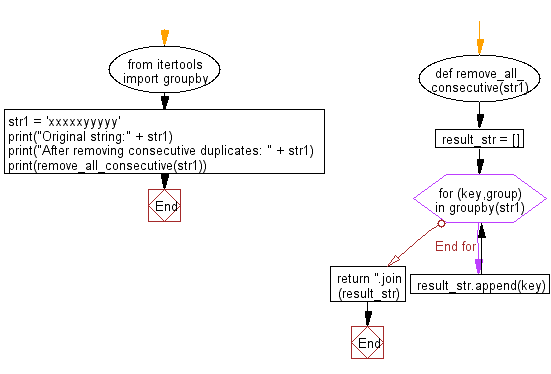
Visual Presentation:
Sample Solution - 2:
Python Code:
# Import itertools
import itertools
# Function to remove consecutive duplicates
def remove_consecutive_duplicates(s1):
# Group into consecutive characters
# Keep only first instance of each
return ''.join(i for i, _ in itertools.groupby(s1))
# Test string
s1= "aabcdaee"
# Print original string
print("Original String: ",s1)
# Print message
print("\nRemoving all consecutive duplicates:")
# Print string with duplicates removed
print(remove_consecutive_duplicates(s1))
Sample Output:
Original String: aabcdaee Removing all consecutive duplicates: abcdae
Flowchart:
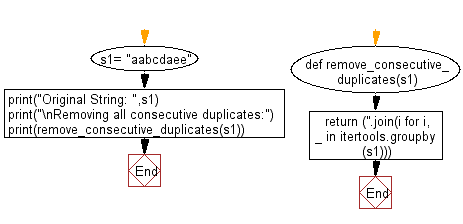
Python Code Editor:
Previous: Write a Python program to make two given strings (lower case, may or may not be of the same length) anagrams removing any characters from any of the strings.
Next: Write a Python program to create two strings from a given string. Create the first string using those character which occurs only once and create the second string which consists of multi-time occurring characters in the said string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics