Python: Make two given strings anagrams removing any characters from any of the strings
Python String: Exercise-66 with Solution
Make strings anagrams (retain characters).
Write a Python program to make two given strings (lower case, may or may not be of the same length) anagrams without removing any characters from any of the strings.
Visual Presentation:
Sample Solution:
Python Code:
# Function to create character map
def make_map(s):
temp_map = {}
for char in s:
if char not in temp_map:
temp_map[char] = 1
else:
temp_map[char] += 1
return temp_map
# Function to calculate edits to make anagram
def make_anagram(str1, str2):
# Create maps for each string
str1_map1 = make_map(str1)
str2_map2 = make_map(str2)
ctr = 0
# Loop through keys in second map
for key in str2_map2.keys():
# If key not in first map, add to counter
if key not in str1_map1:
ctr += str2_map2[key]
# Else add difference
else:
ctr += max(0, str2_map2[key]-str1_map1[key])
# Loop through keys in first map
for key in str1_map1.keys():
# If key not in second map, add to counter
if key not in str2_map2:
ctr += str1_map1[key]
# Else add difference
else:
ctr += max(0, str1_map1[key]-str2_map2[key])
return ctr
# Get input strings
str1 = input("Input string1: ")
str2 = input("Input string2: ")
# Print number of edits
print(make_anagram(str1, str2))
Sample Output:
Input string1: The quick brown fox Input string2: jumps over the lazy dog 24
Flowchart:
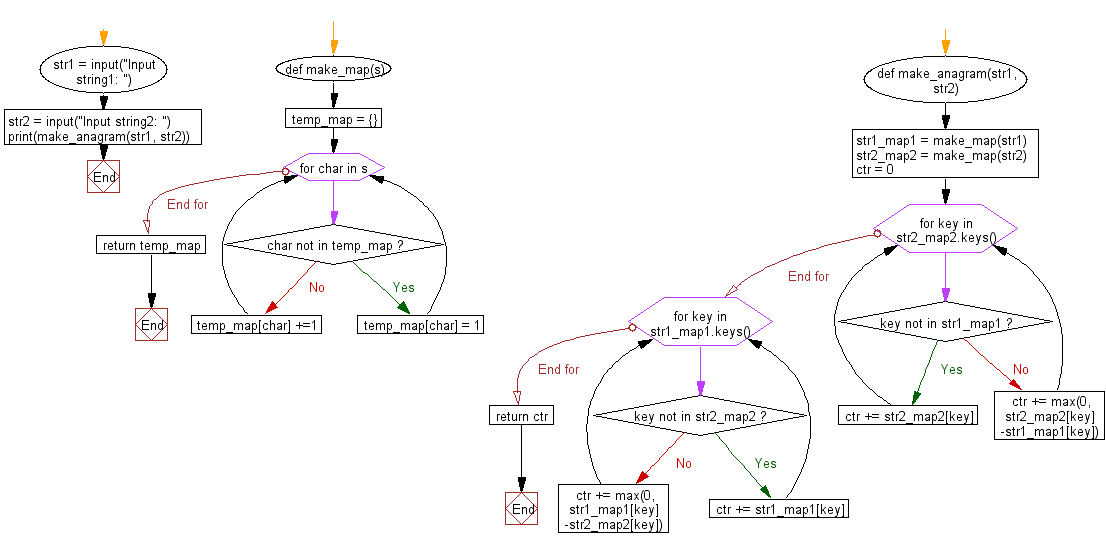
Python Code Editor:
Previous: Write a Python program to find all the common characters in lexicographical order from two given lower case strings. If there are no common letters print “No common characters”.
Next: Write a Python program to remove all consecutive duplicates of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/string/python-data-type-string-exercise-66.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics