Python: Move spaces to the front of a given string
Move spaces to front of string.
Write a Python program to move spaces to the front of a given string.
Pictorial Presentation:
Sample Solution:
Python Code:
# Define a function 'move_Spaces_front' that takes a string 'str1' as input.
def move_Spaces_front(str1):
# Create a list 'noSpaces_char' containing all characters from 'str1' that are not spaces.
noSpaces_char = [ch for ch in str1 if ch != ' ']
# Calculate the number of space characters in the input string 'str1'.
spaces_char = len(str1) - len(noSpaces_char)
# Create a string 'result' containing the same number of space characters at the beginning.
result = ' ' * spaces_char
# Concatenate the 'result' string with the characters in 'noSpaces_char' and enclose it in double quotes.
result = '"' + result + ''.join(noSpaces_char) + '"'
return result # Return the modified string.
# Call the 'move_Spaces_front' function with different input strings and print the results.
print(move_Spaces_front("w3resource . com "))
print(move_Spaces_front(" w3resource.com "))
Sample Output:
" w3resource.com" " w3resource.com"
Flowchart:
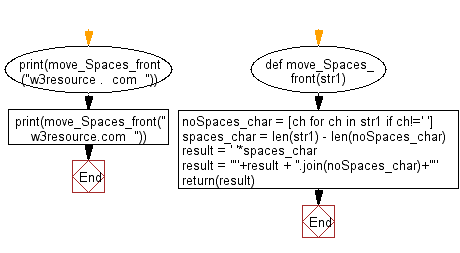
Python Code Editor:
Previous: Write a Python program to remove spaces from a given string.
Next: Write a Python program to find the maximum occuring character in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics