Python: Find the second most repeated word in a given string
Find second most repeated word.
Write a Python program to find the second most repeated word in a given string.
Pictorial Presentation:
Sample Solution:
Python Code:
# Define a function 'word_count' that takes a string 'str' as input.
def word_count(str):
# Create an empty dictionary 'counts' to store word frequencies.
counts = dict()
# Split the input string into words using spaces and store them in the 'words' list.
words = str.split()
# Iterate through each word in the 'words' list.
for word in words:
# Check if the word is already in the 'counts' dictionary.
if word in counts:
counts[word] += 1 # If it is, increment the count.
else:
counts[word] = 1 # If it's not, add it to the dictionary with a count of 1.
# Sort the items in the 'counts' dictionary based on the word frequencies.
# The result is a list of key-value pairs sorted by values.
counts_x = sorted(counts.items(), key=lambda kv: kv[1])
# Return the second-to-last item from the sorted list, which corresponds to the word with the second-highest frequency.
return counts_x[-2]
# Call the 'word_count' function with a long input string and print the result.
print(word_count("Both of these issues are fixed by postponing the evaluation of annotations. Instead of compiling code which executes expressions in annotations at their definition time, the compiler stores the annotation in a string form equivalent to the AST of the expression in question. If needed, annotations can be resolved at runtime using typing.get_type_hints(). In the common case where this is not required, the annotations are cheaper to store (since short strings are interned by the interpreter) and make startup time faster."))
Sample Output:
('of', 4)
Flowchart:
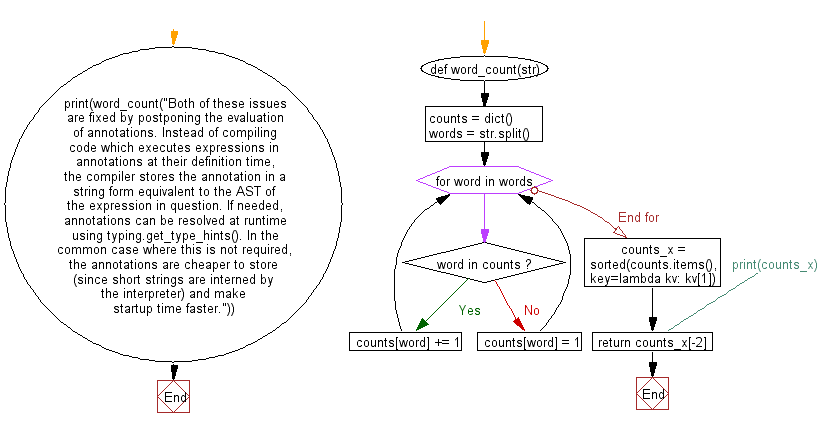
Python Code Editor:
Previous: Write a Python program to find the first repeated word in a given string.
Next:Write a Python program to remove spaces from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics