Python: Find the first repeated character of a given string where the index of first occurrence is smallest
Python String: Exercise-54 with Solution
Find repeated character with smallest index.
Write a Python program to find the first repeated character in a given string where the index of the first occurrence is smallest.
Visual Presentation:
Sample Solution:
Python Code:
# Define a function that finds the first repeated character in a string with the smallest distance between the repetitions.
def first_repeated_char_smallest_distance(str1):
temp = {} # Create an empty dictionary to store characters and their indices.
for ch in str1: # Iterate through each character in the input string.
if ch in temp: # If the character is already in the dictionary (repeated),
return ch, str1.index(ch) # Return the character and its first occurrence index.
else:
temp[ch] = 0 # Add the character to the dictionary with a value of 0 to mark its presence.
return 'None' # If no repeated character is found, return 'None'.
# Test the function with different input strings.
print(first_repeated_char_smallest_distance("abcabc")) # Output: ('a', 0) - 'a' is the first repeated character at index 0.
print(first_repeated_char_smallest_distance("abcb")) # Output: ('b', 1) - 'b' is the first repeated character at index 1.
print(first_repeated_char_smallest_distance("abcc")) # Output: ('c', 1) - 'c' is the first repeated character at index 1.
print(first_repeated_char_smallest_distance("abcxxy")) # Output: ('x', 3) - 'x' is the first repeated character at index 3.
Sample Output:
('a', 0) ('b', 1) ('c', 2) ('x', 3)
Flowchart:
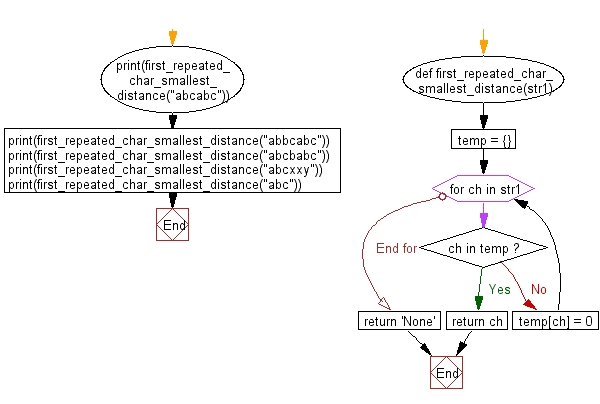
Python Code Editor:
Previous: Write a Python program to find the first repeated character in a given string.
Next:Write a Python program to find the first repeated word in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/string/python-data-type-string-exercise-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics