Python: Find the first repeated character in a given string
Python String: Exercise-53 with Solution
Write a Python program to find the first repeated character in a given string.
Visual Presentation:
Sample Solution:
Python Code:
# Define a function 'first_repeated_char' that takes a string 'str1' as input.
def first_repeated_char(str1):
# Iterate through each character 'c' and its index 'index' in the input string 'str1'.
for index, c in enumerate(str1):
# Check if the count of character 'c' in the substring of 'str1' up to 'index+1' is greater than 1.
if str1[:index + 1].count(c) > 1:
return c # Return the first repeated character found.
return "None" # Return "None" if no repeated character is found.
# Call the 'first_repeated_char' function with different input strings and print the results.
print(first_repeated_char("abcdabcd"))
print(first_repeated_char("abcd"))
Sample Output:
a None
Flowchart:
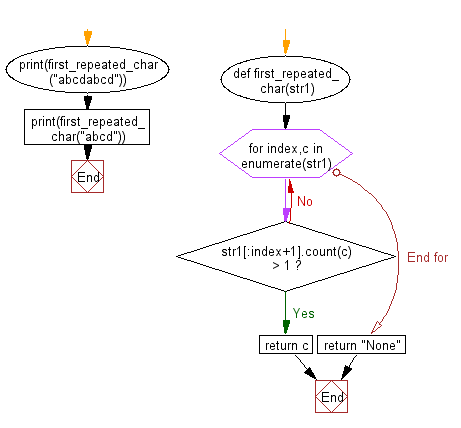
Python Code Editor:
Previous: Write a Python program to print all permutations with given repetition number of characters of a given string.
Next:Write a Python program to find the first repeated character of a given string where the index of first occurrence is smallest.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/string/python-data-type-string-exercise-53.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics