Python: Print all permutations with given repetition number of characters of a given string
Permutations with repetition in string.
Write a Python program to print all permutations with a given repetition number of characters of a given string.
Visual Presentation:
Sample Solution:
Python Code:
# Import the 'product' function from the 'itertools' module.
from itertools import product
# Define a function 'all_repeat' that takes a string 'str1' and an integer 'rno' as input.
def all_repeat(str1, rno):
# Convert the input string 'str1' to a list of characters.
chars = list(str1)
# Create an empty list 'results' to store the combinations of characters.
results = []
# Generate all possible combinations of characters from 'chars' with a length of 'rno'.
for c in product(chars, repeat=rno):
results.append(c)
# Return the list of generated combinations.
return results
# Call the 'all_repeat' function with different inputs and print the results.
print(all_repeat('xyz', 3))
print(all_repeat('xyz', 2))
print(all_repeat('abcd', 4))
Sample Output:
[('x', 'x', 'x'), ('x', 'x', 'y'), ('x', 'x', 'z'), ('x', 'y', 'x'), ('x', 'y', 'y'), ('x', 'y', 'z'), ('x', 'z', 'x'), ('x', 'z', 'y'), ('x', 'z', 'z'), ('y', 'x', 'x'), ('y', 'x', 'y'), ('y', 'x', 'z'), ('y', 'y', 'x'), ('y', 'y', 'y'), ('y', 'y', 'z'), ('y', 'z', 'x'), ('y', 'z', 'y'), ('y', 'z', 'z'), ('z', 'x', 'x'), ('z', 'x', 'y'), ('z', 'x', 'z'), ('z', 'y', 'x'), ('z', 'y', 'y'), ('z', 'y', 'z'), ('z', 'z', 'x'), ('z', 'z', 'y'), ('z', 'z', 'z')] [('x', 'x'), ('x', 'y'), ('x', 'z'), ('y', 'x'), ('y', 'y'), ('y', 'z'), ('z', 'x'), ('z', 'y'), ('z', 'z')] [('a', 'a', 'a', 'a'), ('a', 'a', 'a', 'b'), ('a', 'a', 'a', 'c'), ('a', 'a', 'a', 'd'), ('a', 'a', 'b', 'a'), ('a', 'a', 'b', 'b'), ('a', 'a', 'b', 'c'), ('a', 'a', 'b', 'd'), ('a', 'a', 'c', 'a'), ('a', 'a', 'c', 'b'), ('a', 'a', 'c', 'c'), ('a', 'a', 'c', 'd'), ('a', 'a', 'd', 'a'), ('a', 'a', 'd', 'b'), ('a', 'a', 'd', 'c'), ('a', 'a', 'd', 'd'), ('a', 'b', 'a', 'a'), ('a', 'b', 'a', 'b'), ('a', 'b', 'a', 'c'), ('a', 'b', 'a', 'd'), ('a', 'b', 'b', 'a'), ('a', 'b', 'b', 'b'), ('a', 'b', 'b', 'c'), ('a', 'b', 'b', 'd'), ('a', 'b', 'c', 'a'), ('a', 'b', 'c', 'b'), ('a', 'b', 'c', 'c'), ('a', 'b', 'c', 'd'), ('a', 'b', 'd', 'a'), ('a', 'b', 'd', 'b'), ('a', 'b', 'd', 'c'), ('a', 'b', 'd', 'd'), ('a', 'c', 'a', 'a'), ('a', 'c', 'a', 'b'), ('a', 'c', 'a', 'c'), ('a', 'c', 'a', 'd'), ('a', 'c', 'b', 'a'), ('a', 'c', 'b', 'b'), ('a', 'c', 'b', 'c'), ('a', 'c', 'b', 'd'), ('a', 'c', 'c', 'a'), ('a', 'c', 'c', 'b'), ('a', 'c', 'c', 'c'), ('a', 'c', 'c', 'd'), ('a', 'c', 'd', 'a'), ('a', 'c', 'd', 'b'), ('a', 'c', 'd', 'c'), ('a', 'c', 'd', 'd'), ('a', 'd', 'a', 'a'), ('a', 'd', 'a', 'b'), ('a', 'd', 'a', 'c'), ('a', 'd', 'a', 'd'), ('a', 'd', 'b', 'a'), ('a', 'd', 'b', 'b'), ('a', 'd', 'b', 'c'), ('a', 'd', 'b', 'd'), ('a', 'd', 'c', 'a'), ('a', 'd', 'c', 'b'), ('a', 'd', 'c', 'c'), ('a', 'd', 'c', 'd'), ('a', 'd', 'd', 'a'), ('a', 'd', 'd', 'b'), ('a', 'd', 'd', 'c'), ('a', 'd', 'd', 'd'), ('b', 'a', 'a', 'a'), ('b', 'a', 'a', 'b'), ('b', 'a', 'a', 'c'), ('b', 'a', 'a', 'd'), ('b', 'a', 'b', 'a'), ('b', 'a', 'b', 'b'), ('b', 'a', 'b', 'c'), ('b', 'a', 'b', 'd'), ('b', 'a', 'c', 'a'), ('b', 'a', 'c', 'b'), ('b', 'a', 'c', 'c'), ('b', 'a', 'c', 'd'), ('b', 'a', 'd', 'a'), ('b', 'a', 'd', 'b'), ('b', 'a', 'd', 'c'), ('b', 'a', 'd', 'd'), ('b', 'b', 'a', 'a'), ('b', 'b', 'a', 'b'), ('b', 'b', 'a', 'c'), ('b', 'b', 'a', 'd'), ('b', 'b', 'b', 'a'), ('b', 'b', 'b', 'b'), ('b', 'b', 'b', 'c'), ('b', 'b', 'b', 'd'), ('b', 'b', 'c', 'a'), ('b', 'b', 'c', 'b'), ('b', 'b', 'c', 'c'), ('b', 'b', 'c', 'd'), ('b', 'b', 'd', 'a'), ('b', 'b', 'd', 'b'), ('b', 'b', 'd', 'c'), ('b', 'b', 'd', 'd'), ('b', 'c', 'a', 'a'), ('b', 'c', 'a', 'b'), ('b', 'c', 'a', 'c'), ('b', 'c', 'a', 'd'), ('b', 'c', 'b', 'a'), ('b', 'c', 'b', 'b'), ('b', 'c', 'b', 'c'), ('b', 'c', 'b', 'd'), ('b', 'c', 'c', 'a'), ('b', 'c', 'c', 'b'), ('b', 'c', 'c', 'c'), ('b', 'c', 'c', 'd'), ('b', 'c', 'd', 'a'), ('b', 'c', 'd', 'b'), ('b', 'c', 'd', 'c'), ('b', 'c', 'd', 'd'), ('b', 'd', 'a', 'a'), ('b', 'd', 'a', 'b'), ('b', 'd', 'a', 'c'), ('b', 'd', 'a', 'd'), ('b', 'd', 'b', 'a'), ('b', 'd', 'b', 'b'), ('b', 'd', 'b', 'c'), ('b', 'd', 'b', 'd'), ('b', 'd', 'c', 'a'), ('b', 'd', 'c', 'b'), ('b', 'd', 'c', 'c'), ('b', 'd', 'c', 'd'), ('b', 'd', 'd', 'a'), ('b', 'd', 'd', 'b'), ('b', 'd', 'd', 'c'), ('b', 'd', 'd', 'd'), ('c', 'a', 'a', 'a'), ('c', 'a', 'a', 'b'), ('c', 'a', 'a', 'c'), ('c', 'a', 'a', 'd'), ('c', 'a', 'b', 'a'), ('c', 'a', 'b', 'b'), ('c', 'a', 'b', 'c'), ('c', 'a', 'b', 'd'), ('c', 'a', 'c', 'a'), ('c', 'a', 'c', 'b'), ('c', 'a', 'c', 'c'), ('c', 'a', 'c', 'd'), ('c', 'a', 'd', 'a'), ('c', 'a', 'd', 'b'), ('c', 'a', 'd', 'c'), ('c', 'a', 'd', 'd'), ('c', 'b', 'a', 'a'), ('c', 'b', 'a', 'b'), ('c', 'b', 'a', 'c'), ('c', 'b', 'a', 'd'), ('c', 'b', 'b', 'a'), ('c', 'b', 'b', 'b'), ('c', 'b', 'b', 'c'), ('c', 'b', 'b', 'd'), ('c', 'b', 'c', 'a'), ('c', 'b', 'c', 'b'), ('c', 'b', 'c', 'c'), ('c', 'b', 'c', 'd'), ('c', 'b', 'd', 'a'), ('c', 'b', 'd', 'b'), ('c', 'b', 'd', 'c'), ('c', 'b', 'd', 'd'), ('c', 'c', 'a', 'a'), ('c', 'c', 'a', 'b'), ('c', 'c', 'a', 'c'), ('c', 'c', 'a', 'd'), ('c', 'c', 'b', 'a'), ('c', 'c', 'b', 'b'), ('c', 'c', 'b', 'c'), ('c', 'c', 'b', 'd'), ('c', 'c', 'c', 'a'), ('c', 'c', 'c', 'b'), ('c', 'c', 'c', 'c'), ('c', 'c', 'c', 'd'), ('c', 'c', 'd', 'a'), ('c', 'c', 'd', 'b'), ('c', 'c', 'd', 'c'), ('c', 'c', 'd', 'd'), ('c', 'd', 'a', 'a'), ('c', 'd', 'a', 'b'), ('c', 'd', 'a', 'c'), ('c', 'd', 'a', 'd'), ('c', 'd', 'b', 'a'), ('c', 'd', 'b', 'b'), ('c', 'd', 'b', 'c'), ('c', 'd', 'b', 'd'), ('c', 'd', 'c', 'a'), ('c', 'd', 'c', 'b'), ('c', 'd', 'c', 'c'), ('c', 'd', 'c', 'd'), ('c', 'd', 'd', 'a'), ('c', 'd', 'd', 'b'), ('c', 'd', 'd', 'c'), ('c', 'd', 'd', 'd'), ('d', 'a', 'a', 'a'), ('d', 'a', 'a', 'b'), ('d', 'a', 'a', 'c'), ('d', 'a', 'a', 'd'), ('d', 'a', 'b', 'a'), ('d', 'a', 'b', 'b'), ('d', 'a', 'b', 'c'), ('d', 'a', 'b', 'd'), ('d', 'a', 'c', 'a'), ('d', 'a', 'c', 'b'), ('d', 'a', 'c', 'c'), ('d', 'a', 'c', 'd'), ('d', 'a', 'd', 'a'), ('d', 'a', 'd', 'b'), ('d', 'a', 'd', 'c'), ('d', 'a', 'd', 'd'), ('d', 'b', 'a', 'a'), ('d', 'b', 'a', 'b'), ('d', 'b', 'a', 'c'), ('d', 'b', 'a', 'd'), ('d', 'b', 'b', 'a'), ('d', 'b', 'b', 'b'), ('d', 'b', 'b', 'c'), ('d', 'b', 'b', 'd'), ('d', 'b', 'c', 'a'), ('d', 'b', 'c', 'b'), ('d', 'b', 'c', 'c'), ('d', 'b', 'c', 'd'), ('d', 'b', 'd', 'a'), ('d', 'b', 'd', 'b'), ('d', 'b', 'd', 'c'), ('d', 'b', 'd', 'd'), ('d', 'c', 'a', 'a'), ('d', 'c', 'a', 'b'), ('d', 'c', 'a', 'c'), ('d', 'c', 'a', 'd'), ('d', 'c', 'b', 'a'), ('d', 'c', 'b', 'b'), ('d', 'c', 'b', 'c'), ('d', 'c', 'b', 'd'), ('d', 'c', 'c', 'a'), ('d', 'c', 'c', 'b'), ('d', 'c', 'c', 'c'), ('d', 'c', 'c', 'd'), ('d', 'c', 'd', 'a'), ('d', 'c', 'd', 'b'), ('d', 'c', 'd', 'c'), ('d', 'c', 'd', 'd'), ('d', 'd', 'a', 'a'), ('d', 'd', 'a', 'b'), ('d', 'd', 'a', 'c'), ('d', 'd', 'a', 'd'), ('d', 'd', 'b', 'a'), ('d', 'd', 'b', 'b'), ('d', 'd', 'b', 'c'), ('d', 'd', 'b', 'd'), ('d', 'd', 'c', 'a'), ('d', 'd', 'c', 'b'), ('d', 'd', 'c', 'c'), ('d', 'd', 'c', 'd'), ('d', 'd', 'd', 'a'), ('d', 'd', 'd', 'b'), ('d', 'd', 'd', 'c'), ('d', 'd', 'd', 'd')]
Flowchart:
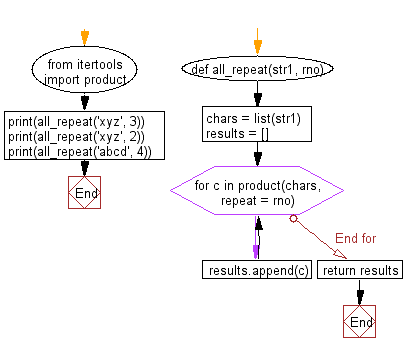
For more Practice: Solve these Related Problems:
- Write a Python program to generate all permutations of a given string where each character can repeat up to a specified number of times.
- Write a Python program to compute the set of unique permutations of a string with allowed repetition using recursion.
- Write a Python program to use itertools.product to simulate permutations with repetition for a given string and repetition count.
- Write a Python program to generate all possible arrangements of characters in a string where each letter appears exactly r times using nested loops.
Go to:
Previous: Write a Python program to find the first non-repeating character in given string.
Next:Write a Python program to find the first repeated character in a given string.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.