Python: Convert a given string into a list of words
Convert string to list of words.
Write a Python program to convert a given string into a list of words.
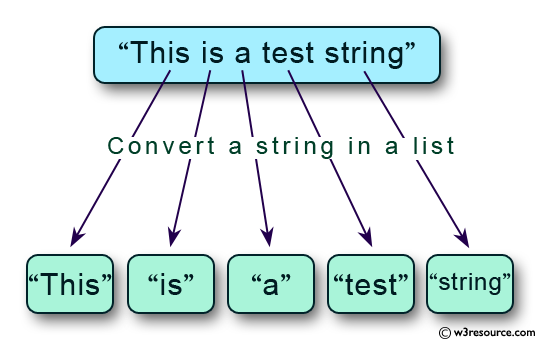
Sample Solution-1:
Python Code:
# Define a string 'str1'.
str1 = "The quick brown fox jumps over the lazy dog."
# Split the string into a list of words using the space character as the separator and print the result.
print(str1.split(' '))
# Update the string 'str1'.
str1 = "The-quick-brown-fox-jumps-over-the-lazy-dog."
# Split the updated string into a list of words using the hyphen character as the separator and print the result.
print(str1.split('-'))
Sample Output:
['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog.'] ['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog.']
Sample Solution-2:
- Use re.findall() with the supplied pattern to find all matching substrings.
- Omit the second argument to use the default regexp, which matches alphanumeric and hyphens.
Python Code:
# Import the 're' module to work with regular expressions.
import re
# Define a function 'string_to_list' that takes a string 's' and an optional 'pattern' as input.
# The 'pattern' parameter defines a regular expression pattern for matching substrings.
def string_to_list(s, pattern='[a-zA-Z-]+'):
# Use the 're.findall' function to find all substrings in 's' that match the 'pattern'.
return re.findall(pattern, s)
# Call the 'string_to_list' function with different input strings and 'pattern' values.
print(string_to_list('The quick brown fox jumps over the lazy dog.'))
print(string_to_list('Python, JavaScript & C++'))
print(string_to_list('build -q --out one-item', r'\b[a-zA-Z-]+\b'))
Sample Output:
['The', 'quick', 'brown', 'fox', 'jumps', 'over', 'the', 'lazy', 'dog'] ['Python', 'JavaScript', 'C'] ['build', 'q', 'out', 'one-item']
Flowchart:
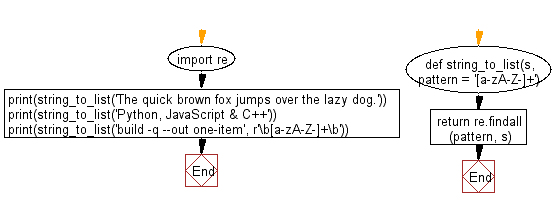
For more Practice: Solve these Related Problems:
- Write a Python program to split a sentence into a list of words using the split() method.
- Write a Python program to use regular expressions to convert a string into a list of words, ignoring punctuation.
- Write a Python program to implement a function that returns a list of words from a given string.
- Write a Python program to strip extra whitespace and then convert the input string into a list of words.
Python Code Editor:
Previous: Write a Python program to check if a string contains all letters of the alphabet.
Next: Write a Python program to lowercase first n characters in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics