Python: Check whether a string contains all letters of the alphabet
Check if string has all alphabet letters.
Write a Python program to check whether a string contains all letters of the alphabet.
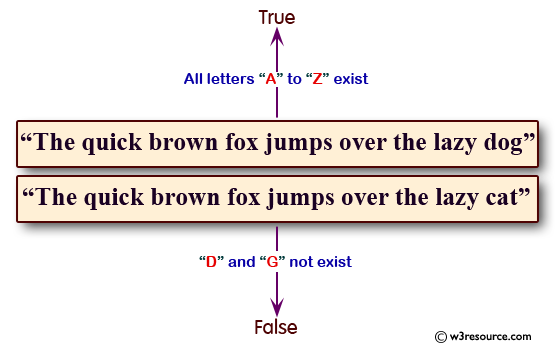
Sample Solution:
Python Code:
# Import the 'string' module to access the lowercase alphabet.
import string
# Create a set 'alphabet' containing all lowercase letters using 'string.ascii_lowercase'.
alphabet = set(string.ascii_lowercase)
# Define an input string.
input_string = 'The quick brown fox jumps over the lazy dog'
# Check if the set of lowercase characters in the input string contains all the letters of the alphabet.
# Print the result (True or False).
print(set(input_string.lower()) >= alphabet)
# Update the input string.
input_string = 'The quick brown fox jumps over the lazy cat'
# Check if the set of lowercase characters in the updated input string contains all the letters of the alphabet.
# Print the result (True or False).
print(set(input_string.lower()) >= alphabet)
Sample Output:
True False
Flowchart:
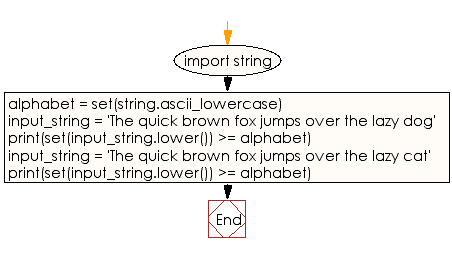
Python Code Editor:
Previous: Write a Python program to print the index of the character in a string.
Next: Write a Python program to convert a given string into a list of words.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics