Python: Format a number with a percentage
Format number as percentage.
Write a Python program to format a number with a percentage.
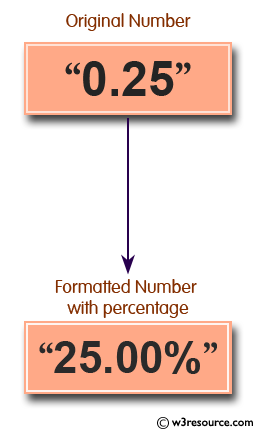
Sample Solution:
Python Code:
Sample Output:
Original Number: 0.25 Formatted Number with percentage: 25.00% Original Number: -0.25 Formatted Number with percentage: -25.00%
Flowchart:
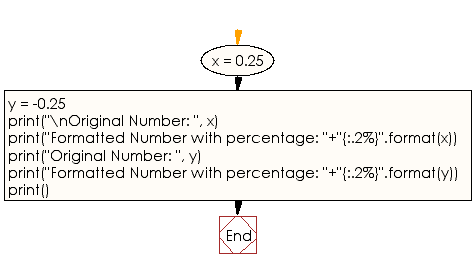
For more Practice: Solve these Related Problems:
- Write a Python program to convert a decimal number into a percentage format with two decimal places.
- Write a Python program to use f-string formatting to display a float as a percentage with a '%' symbol appended.
- Write a Python program to implement a function that multiplies a decimal by 100 and formats it as a percentage.
- Write a Python program to use the format() function to output a number in percentage format with specified precision.
Python Code Editor:
Previous: Write a Python program to display a number with a comma separator.
Next: Write a Python program to display a number in left, right and center aligned of width 10.
What is the difficulty level of this exercise?
Based on 544 votes, average difficulty level of this exercise is Medium
.
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics