Python: Sort a string lexicographically
Python String: Exercise-22 with Solution
Write a Python program to sort a string lexicographically.
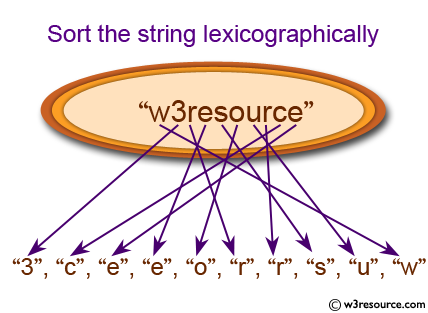
Sample Solution:
Python Code:
# Define a function named lexicographic_sort that takes one argument, 's'.
def lexicographic_sort(s):
# Use a nested sorting approach:
# 1. First, sort the characters of the string 's' in ascending order.
# 2. Then, sort the sorted characters based on their uppercase representations (case-insensitive).
return sorted(sorted(s), key=str.upper)
# Call the lexicographic_sort function with different input strings and print the results.
print(lexicographic_sort('w3resource')) # Output: '3ceeorrsuw'
print(lexicographic_sort('quickbrown')) # Output: 'biknqorwuc'
Sample Output:
['3', 'c', 'e', 'e', 'o', 'r', 'r', 's', 'u', 'w'] ['b', 'c', 'i', 'k', 'n', 'o', 'q', 'r', 'u', 'w']
Python Code Editor:
Previous: Write a Python function to convert a given string to all uppercase if it contains at least 2 uppercase characters in the first 4 characters.
Next: Write a Python program to remove a newline in Python.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/string/python-data-type-string-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics