Python: Insert a string in the middle of a string
Insert string into middle of another.
Write a Python function to insert a string in the middle of a string.
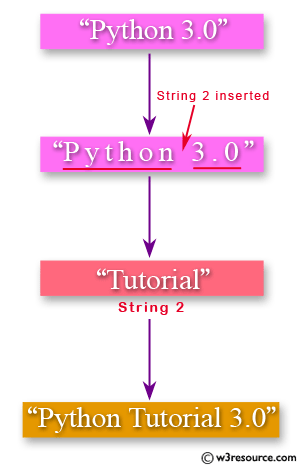
Sample Solution:
Python Code:
# Define a function named insert_string_middle that takes two arguments, 'str' and 'word'.
def insert_string_middle(str, word):
# Create and return a new string by concatenating the first two characters of 'str',
# followed by the 'word', and then the remaining characters of 'str' starting from the third character.
return str[:2] + word + str[2:]
# Call the insert_string_middle function with different input strings and words and print the results.
print(insert_string_middle('[[]]', 'Python')) # Output: '[Python]'
print(insert_string_middle('{{}}', 'PHP')) # Output: '{{PHP}}'
print(insert_string_middle('<<>>', 'HTML')) # Output: '<>'
Sample Output:
[[Python]] {{PHP}} <<HTML>>
Flowchart:
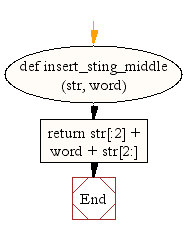
For more Practice: Solve these Related Problems:
- Write a Python program to insert one string into the middle of another string using slicing.
- Write a Python function that calculates the midpoint of a string and inserts a given substring at that position.
- Write a Python program to merge two strings by placing the second string into the center of the first string.
- Write a Python program to implement this functionality recursively, dividing the string into two halves and inserting the new string.
Python Code Editor:
Previous: Write a Python function to create the HTML string with tags around the word(s).
Next: Write a Python function to get a string made of 4 copies of the last two characters of a specified string (length must be at least 2).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics