Python Exercises: Count the number of leap years within the range
Python String: Exercise-109 with Solution
Write a Python program that counts the number of leap years within the range of years. Ranges of years should be accepted as strings.
Sample Data:
("1981-1991") -> 2
("2000-2020") -> 6
Sample Solution-1:
Python Code:
# Define a function to count the number of leap years within a given range of years
def test(r_years):
# Extract the start and end year from the input string
start_year, end_year = map(int, r_years.split('-'))
# Use a generator expression to count the leap years within the specified range
return sum(is_leap_year(year) for year in range(start_year, end_year+1))
# Define a function to check if a given year is a leap year
def is_leap_year(y):
# Check the leap year conditions and return True if it's a leap year, otherwise False
if y % 400 == 0:
return True
if y % 100 == 0:
return False
if y % 4 == 0:
return True
else:
return False
# Initialize a string representing a range of years
text = "1981-1991"
# Print the range of years
print("Range of years:", text)
# Print a message indicating the counting of leap years within the specified range
print("Count the number of leap years within the said range:")
# Call the function to count and print the result
print(test(text))
# Repeat the process with a different range of years
text = "2000-2020"
# Print the range of years
print("\nRange of years:", text)
# Print a message indicating the counting of leap years within the specified range
print("Count the number of leap years within the said range:")
# Call the function to count and print the result
print(test(text))
Sample Output:
Range of years: 1981-1991 Count the number of leap years within the said range: 2 Range of years: 2000-2020 Count the number of leap years within the said range: 6
Flowchart:
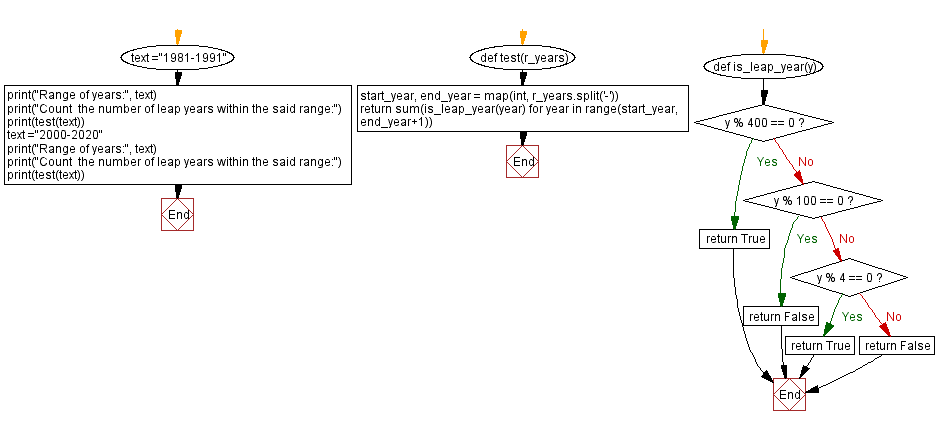
Sample Solution-2:
Python Code:
# Define a function to count the number of leap years within a given range of years
def test(r_years):
# Convert the input range of years string into a list of integers
r = [int(x) for x in r_years.split('-')]
# Use a list comprehension to create a list of leap years within the specified range
return [is_leap_year(int(x)) for x in range(r[0], r[1] + 1)].count(True)
# Define a function to check if a given year is a leap year
def is_leap_year(y):
# Check the leap year conditions and return True if it's a leap year, otherwise False
if y % 400 == 0:
return True
if y % 100 == 0:
return False
if y % 4 == 0:
return True
else:
return False
# Initialize a string representing a range of years
text = "1981-1991"
# Print the range of years
print("Range of years:", text)
# Print a message indicating the counting of leap years within the specified range
print("Count the number of leap years within the said range:")
# Call the function to count and print the result
print(test(text))
# Repeat the process with a different range of years
text = "2000-2020"
# Print the range of years
print("\nRange of years:", text)
# Print a message indicating the counting of leap years within the specified range
print("Count the number of leap years within the said range:")
# Call the function to count and print the result
print(test(text))
Sample Output:
Range of years: 1981-1991 Count the number of leap years within the said range: 2 Range of years: 2000-2020 Count the number of leap years within the said range: 6
Flowchart:
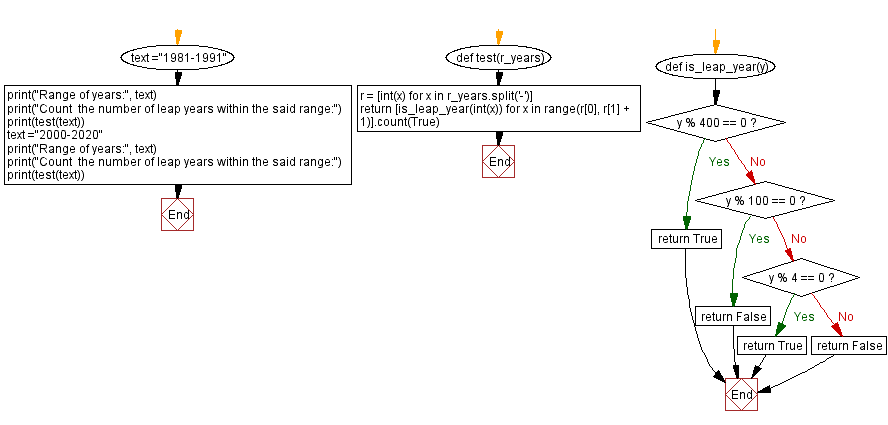
Python Code Editor:
Previous Python Exercise: Hash elements.
Next Python Exercise: Insert space before capital letters in word.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/string/python-data-type-string-exercise-109.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics