Python Exercises: Hash elements
Add hashes around non-vowels.
Write a Python program that takes a string and returns # on both sides of each element, which are not vowels.
Sample Data:
("Green" -> "-G--r-ee-n-"
("White") -> "-W--h-i-t-e"
(“aeiou") -> "aeiou"
Sample Solution-1:
Python Code:
# Define a function to insert hash elements on both sides of each element,
# which are not vowels, in the input string
def test(text):
# Use a list comprehension to create a list of modified elements
return "".join([el, '-' + el + '-'][el not in "AEIOUaeiou"] for el in text)
# Initialize a string
text = "Green"
# Print the original string
print("Original string:", text)
# Print a message indicating the insertion of hash elements
print("Insert Hash elements on both sides of each element, which are not vowels:")
# Call the function to modify the string and print the result
print(test(text))
# Repeat the process with a different string
text = "White"
# Print the original string
print("\nOriginal string:", text)
# Print a message indicating the insertion of hash elements
print("Insert Hash elements on both sides of each element, which are not vowels:")
# Call the function to modify the string and print the result
print(test(text))
# Repeat the process with a string containing only vowels
text = "aeiou"
# Print the original string
print("\nOriginal string:", text)
# Print a message indicating the insertion of hash elements
print("Insert Hash elements on both sides of each element, which are not vowels:")
# Call the function to modify the string and print the result
print(test(text))
Sample Output:
Original string: Green Insert Hash elements on both side of each element, which are not vowels: -G--r-ee-n- Original string: White Insert Hash elements on both side of each element, which are not vowels: -W--h-i-t-e Original string: aeiou Insert Hash elements on both side of each element, which are not vowels: aeiou
Flowchart:
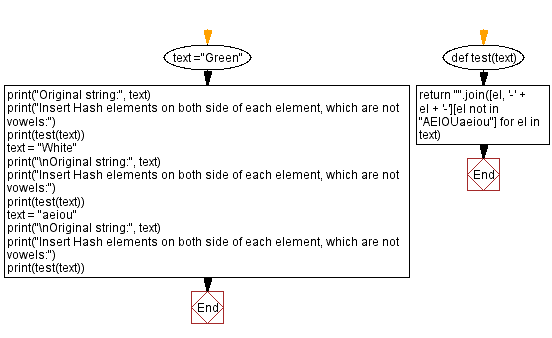
Sample Solution-2:
Python Code:
# Define a function to insert hash elements on both sides of each element in the input string
# if the element is not a vowel, and return the modified string
def test(text):
# Use a generator expression and join to create the modified string
return ''.join('-{}-'.format(el) if el.lower() not in 'aeiou' else el for el in text)
# Initialize a string
text = "Green"
# Print the original string
print("Original string:", text)
# Print a message indicating the insertion of hash elements
print("Insert Hash elements on both sides of each element, which are not vowels:")
# Call the function to modify the string and print the result
print(test(text))
# Repeat the process with a different string
text = "White"
# Print the original string
print("\nOriginal string:", text)
# Print a message indicating the insertion of hash elements
print("Insert Hash elements on both sides of each element, which are not vowels:")
# Call the function to modify the string and print the result
print(test(text))
# Repeat the process with a string containing only vowels
text = "aeiou"
# Print the original string
print("\nOriginal string:", text)
# Print a message indicating the insertion of hash elements
print("Insert Hash elements on both sides of each element, which are not vowels:")
# Call the function to modify the string and print the result
print(test(text))
Sample Output:
Original string: Green Insert Hash elements on both side of each element, which are not vowels: -G--r-ee-n- Original string: White Insert Hash elements on both side of each element, which are not vowels: -W--h-i-t-e Original string: aeiou Insert Hash elements on both side of each element, which are not vowels: aeiou
Flowchart:
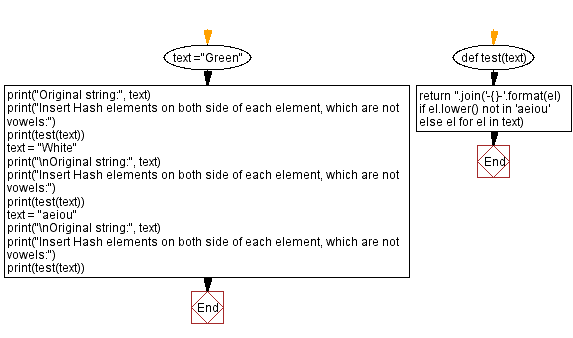
For more Practice: Solve these Related Problems:
- Write a Python program to iterate over a string and wrap non-vowel characters with '#' while leaving vowels unchanged.
- Write a Python program to use a loop and conditional checks to add '#' on both sides of each non-vowel in a string.
- Write a Python program to implement this transformation using list comprehension to surround consonants with hash characters.
- Write a Python program to replace each non-vowel character with a formatted string that includes '#' before and after the character.
Go to:
Previous Python Exercise: Two strings contain three letters at the same index.
Next Python Exercise: Count the number of leap years within the range.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.