Python program to retrieve table information from database
Python SQLAlchemy: Exercise-3 with Solution
Write a Python program that retrieves a student's information from the `students` table using their id.
Sample Solution:
Code:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_base
# Create a SQLite database named students.db
engine = create_engine('sqlite:///testdatabase.db', echo=False)
# Create a base class for declarative models
Base = declarative_base()
# Define the Student model
class Student(Base):
__tablename__ = 'students'
id = Column(Integer, primary_key=True)
studentname = Column(String, nullable=False)
email = Column(String, nullable=False)
# Create a session to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
def get_student_info(student_id):
student = session.query(Student).filter_by(id=student_id).first()
return student
# Retrieve a user's information using their ID
student_id = 22 # Replace with the desired student ID
student = get_student_info(student_id)
if student:
print(f"Student ID: {student.id}")
print(f"Student Name: {student.studentname}")
print(f"Email: {student.email}")
else:
print(f"Student with ID {student_id} not found")
# Close the session
session.close()
Output:
Student ID: 22 Student Name: Hughie Glauco Email: [email protected]
Flowchart:
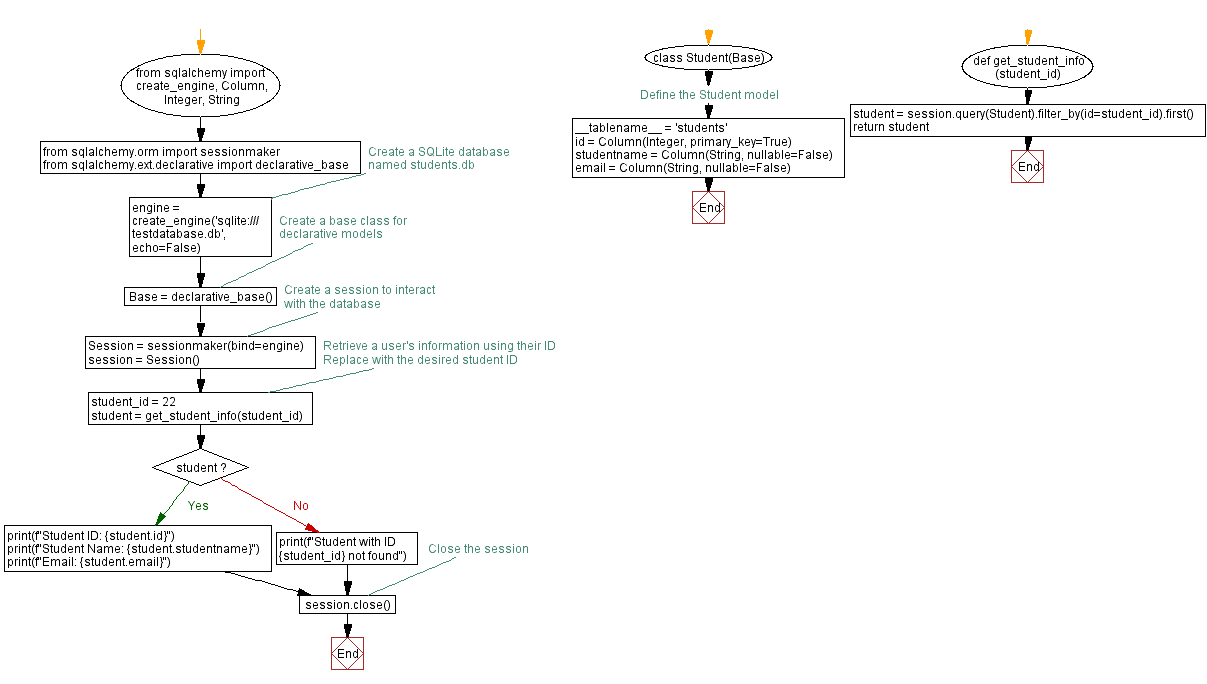
Previous: Python Program: Add new records to database table.
Next: Python program to update a table field.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics