Python: Iteration over sets
2. Iterate Over Sets
Write a Python program to iterate over sets.
Sample Solution-1:
Iterate over the set without indexes:
Python Code:
# Create a set containing the numbers 0 to 5:
num_set = set([0, 1, 2, 3, 4, 5])
# Iterate through the elements in 'num_set' and print them with a space separator:
for n in num_set:
print(n, end=' ')
# Print two newline characters for separation:
print("\n\nCreating a set using string:")
# Create a set 'char_set' using a string, which will create a set of unique characters from the string "w3resource":
char_set = set("w3resource")
# Iterate through the elements in 'char_set' and print them with a space separator:
for val in char_set:
print(val, end=' ')
Sample Output:
0 1 2 3 4 5 Creating a set using string: c e 3 u r o w s
Flowchart:
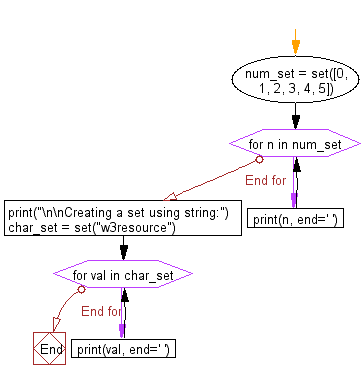
Sample Solution-2:
Iterating over a set as indexed list:
Python Code:
# Create a set 'char_set' by converting the string "w3resource" into a set, which will contain unique characters:
char_set = set("w3resource")
# Convert the 'char_set' back to a list, so it becomes a list of unique characters:
char_set = list(char_set)
# Iterate over the elements of 'char_set' using their index positions in the list:
# 'id' is used as the loop variable to represent the index.
for id in range(len(char_set)):
# Print the character at the current index, separated by a space, using 'end' parameter:
print(char_set[id], end=' ')
Sample Output:
3 w o u e r s c
Flowchart:
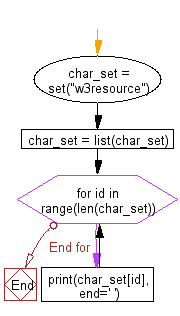
For more Practice: Solve these Related Problems:
- Write a Python program to iterate over a set of strings and print each element in uppercase.
- Write a Python program to loop through a set of numbers and compute the sum of its elements.
- Write a Python program to use a for-loop to display each element of a set alongside its type.
- Write a Python program to iterate over a set and create a new set that contains the square of each numeric element.
Sample Solution-3:
Using the enumerate type:
Python Code:
# Create a set 'num_set' containing the numbers 0 to 5 using set literal:
num_set = {0, 1, 2, 3, 4, 5}
# Iterate over the elements in 'num_set' and use 'enumerate' to get both the index and value:
# 'value' represents the element, and 'char' represents the index in this context.
for value, char in enumerate(num_set):
# Print the element (char) with a space separator, using 'end' parameter:
print(char, end=' ')
Sample Output:
0 1 2 3 4 5
Flowchart:
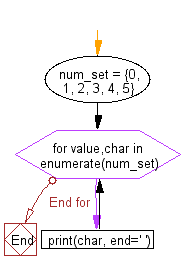
Python Code Editor:
Previous: Write a Python program to create a set.
Next: Write a Python program to add member(s) in a set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.