Python: Find the elements in a given set that are not in another set
Python sets: Exercise-19 with Solution
Write a Python program to find elements in a given set that are not in another set.
Visual Presentation:
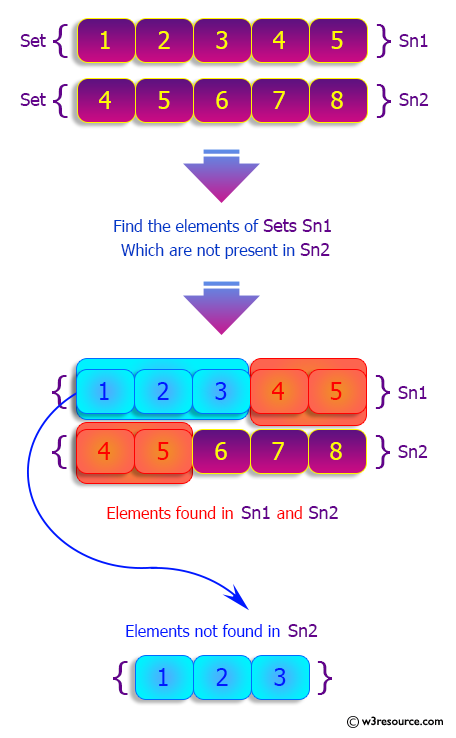
Sample Solution:
Python Code:
# Create sets 'sn1' and 'sn2' with different elements.
sn1 = {1, 2, 3, 4, 5}
sn2 = {4, 5, 6, 7, 8}
# Print a message to indicate the original sets.
print("Original sets:")
print(sn1)
print(sn2)
# Print a message to find the difference between 'sn1' and 'sn2' using the 'difference()' method.
print("Difference of sn1 and sn2 using difference():")
# Use the 'difference()' method to find and print the elements that are in 'sn1' but not in 'sn2'.
print(sn1.difference(sn2))
# Print a message to find the difference between 'sn2' and 'sn1' using the 'difference()' method.
print("Difference of sn2 and sn1 using difference():")
# Use the 'difference()' method to find and print the elements that are in 'sn2' but not in 'sn1'.
print(sn2.difference(sn1))
# Print a message to find the difference between 'sn1' and 'sn2' using the '-' operator.
print("Difference of sn1 and sn2 using - operator:")
# Use the '-' operator to find and print the elements that are in 'sn1' but not in 'sn2'.
print(sn1 - sn2)
# Print a message to find the difference between 'sn2' and 'sn1' using the '-' operator.
print("Difference of sn2 and sn1 using - operator:")
# Use the '-' operator to find and print the elements that are in 'sn2' but not in 'sn1'.
print(sn2 - sn1)
Sample Output:
Original sets: {1, 2, 3, 4, 5} {4, 5, 6, 7, 8} Difference of sn1 and sn2 using difference(): {1, 2, 3} Difference of sn2 and sn1 using difference(): {8, 6, 7} Difference of sn1 and sn2 using - operator: {1, 2, 3} Difference of sn2 and sn1 using - operator: {8, 6, 7}
Python Code Editor:
Previous: Write a Python program to check if a given set is superset of itself and superset of another given set.
Next: Write a Python program to remove the intersection of a 2nd set from the 1st set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics