Python: Reads a given expression and evaluates it
Write a Python program that reads a given expression and evaluates it.
Terms and conditions:
The expression consists of numerical values, operators and parentheses, and the ends with '='.
The operators includes +, -, *, / where, represents, addition, subtraction, multiplication and division.
When two operators have the same precedence, they are applied to left to right.
You may assume that there is no division by zero.
All calculation is performed as integers, and after the decimal point should be truncated
Length of the expression will not exceed 100.
-1 × 10 9 ≤ intermediate results of computation ≤ 10 9
Sample Solution:
Python Code:
#https://bit.ly/2lxQysi
import re
print("Input number of data sets:")
class c(int):
def __add__(self,n):
return c(int(self)+int(n))
def __sub__(self,n):
return c(int(self)-int(n))
def __mul__(self,n):
return c(int(self)*int(n))
def __truediv__(self,n):
return c(int(int(self)/int(n)))
for _ in range(int(input())):
print("Input an expression:")
print(eval(re.sub(r'(\d+)',r'c(\1)',input()[:-1])))
Sample Output:
Input number of data sets: 2 Input an expression: 4-2*3= -2 Input an expression: 4*(8+4+3)= 60
Flowchart:
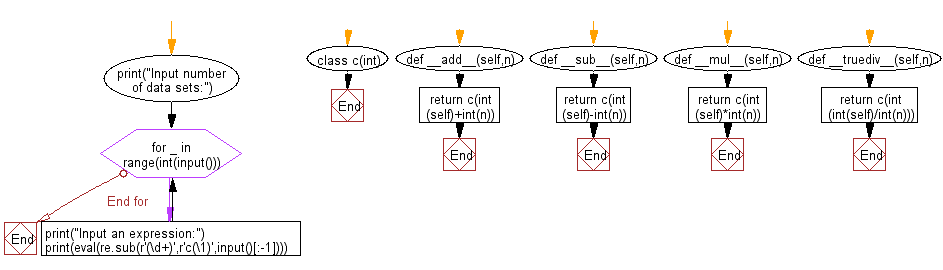
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to insert spaces between words starting with capital letters.
Next: Write a Python program to remove lowercase substrings from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics