Python: Matches a word at the end of a string, with optional punctuation
11. Word at End
Write a Python program that matches a word at the end of a string, with optional punctuation.
Sample Solution:
Python Code:
import re
def text_match(text):
patterns = '\w+\S*$'
if re.search(patterns, text):
return 'Found a match!'
else:
return('Not matched!')
print(text_match("The quick brown fox jumps over the lazy dog."))
print(text_match("The quick brown fox jumps over the lazy dog. "))
print(text_match("The quick brown fox jumps over the lazy dog "))
Sample Output:
Found a match! Not matched! Not matched!
Pictorial Presentation:
Flowchart:
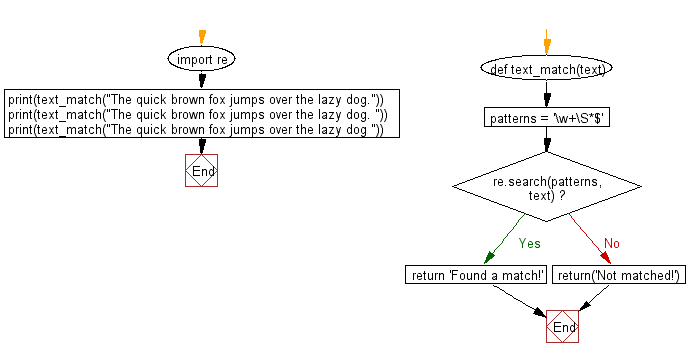
For more Practice: Solve these Related Problems:
- Write a Python program to check if a string ends with a specific word, allowing for optional punctuation.
- Write a Python script to extract the last word of a sentence even if it ends with punctuation.
- Write a Python program to match a pattern that confirms a string’s ending word, regardless of trailing punctuation.
- Write a Python program to verify if the final word in a string matches a given pattern and output its position.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program that matches a word at the beginning of a string.
Next: Write a Python program that matches a word containing 'z'.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.