Python: Check that a string contains only a certain set of characters
Python Regular Expression: Exercise-1 with Solution
Write a Python program to check that a string contains only a certain set of characters (in this case a-z, A-Z and 0-9).
Sample Solution:
Python Code:
import re
def is_allowed_specific_char(string):
charRe = re.compile(r'[^a-zA-Z0-9]')
string = charRe.search(string)
return not bool(string)
print(is_allowed_specific_char("ABCDEFabcdef123450"))
print(is_allowed_specific_char("*&%@#!}{"))
Sample Output:
True False
Pictorial Presentation:
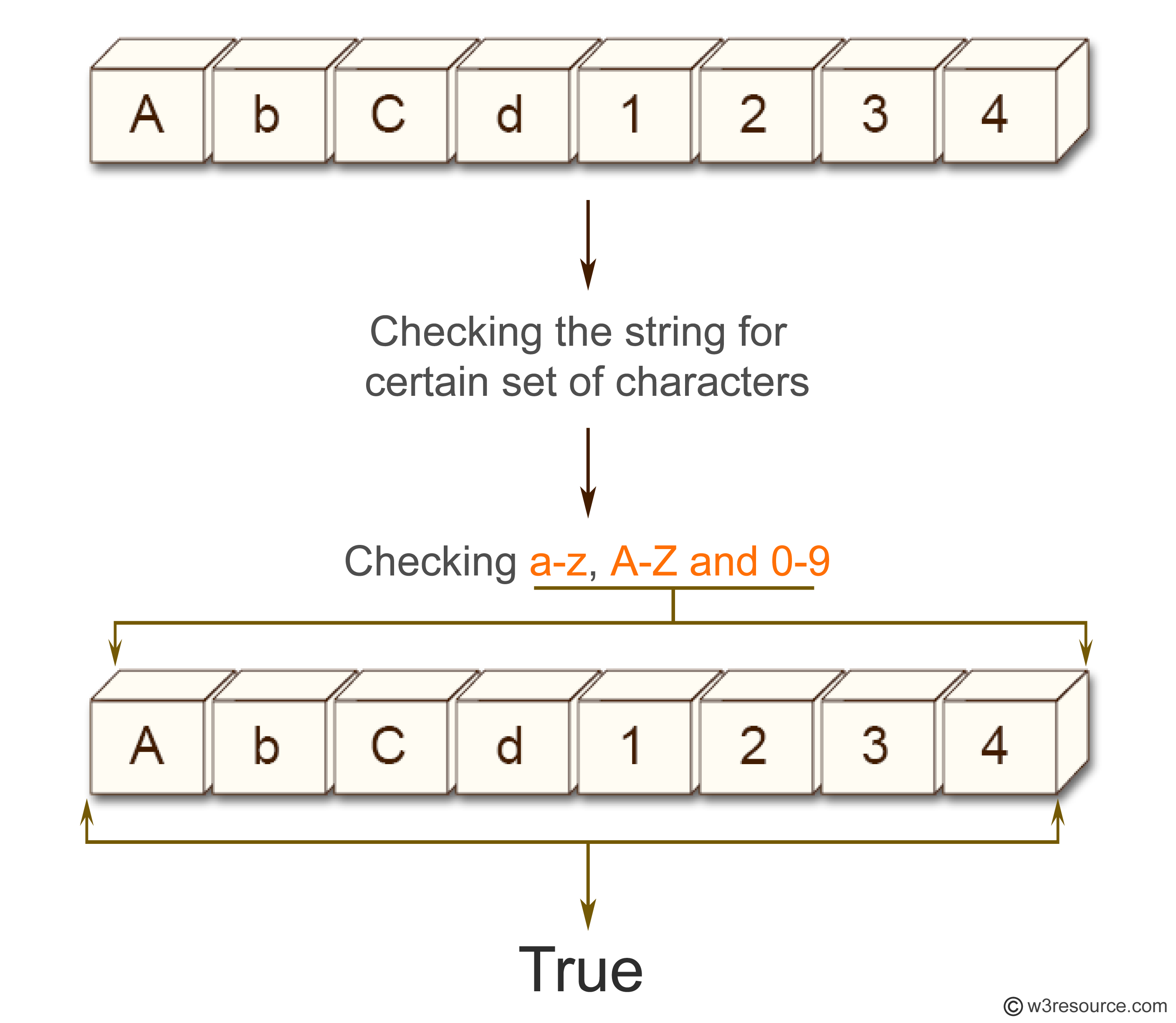
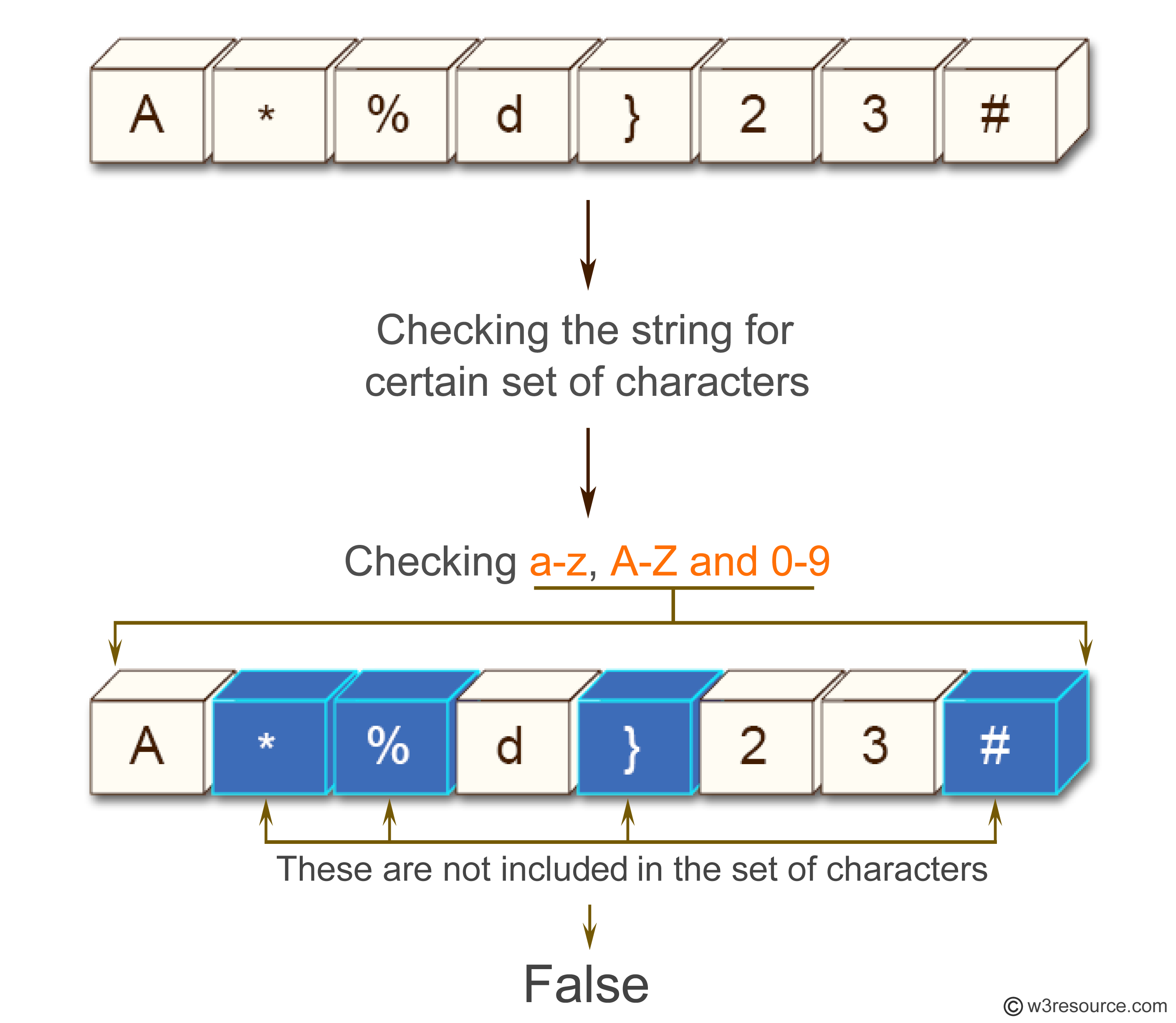
Flowchart:
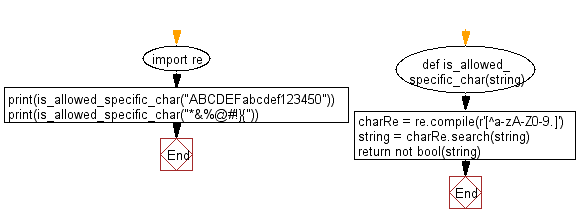
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Regular Expression Home.
Next: Write a Python program that matches a string that has an a followed by zero or more b's.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/re/python-re-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics