Python Exercise: Calculate the number of upper / lower case letters in a string
Python Functions: Exercise-7 with Solution
Write a Python function that accepts a string and counts the number of upper and lower case letters.
Sample Solution:
Python Code:
# Define a function named 'string_test' that counts the number of upper and lower case characters in a string 's'
def string_test(s):
# Create a dictionary 'd' to store the count of upper and lower case characters
d = {"UPPER_CASE": 0, "LOWER_CASE": 0}
# Iterate through each character 'c' in the string 's'
for c in s:
# Check if the character 'c' is in upper case
if c.isupper():
# If 'c' is upper case, increment the count of upper case characters in the dictionary
d["UPPER_CASE"] += 1
# Check if the character 'c' is in lower case
elif c.islower():
# If 'c' is lower case, increment the count of lower case characters in the dictionary
d["LOWER_CASE"] += 1
else:
# If 'c' is neither upper nor lower case (e.g., punctuation, spaces), do nothing
pass
# Print the original string 's'
print("Original String: ", s)
# Print the count of upper case characters
print("No. of Upper case characters: ", d["UPPER_CASE"])
# Print the count of lower case characters
print("No. of Lower case Characters: ", d["LOWER_CASE"])
# Call the 'string_test' function with the input string 'The quick Brown Fox'
string_test('The quick Brown Fox')
Sample Output:
Original String : The quick Brow Fox No. of Upper case characters : 3 No. of Lower case Characters : 13
Explanation:
In the exercise above the code defines a function named "string_test()" that counts the number of upper case and lower case characters in the given string 's'. It utilizes a dictionary to store the counts and then prints the original string along with the counts of upper and lower case characters. Finally, it demonstrates the function by calling it with the input string 'The quick Brown Fox'.
Pictorial presentation:
Flowchart:
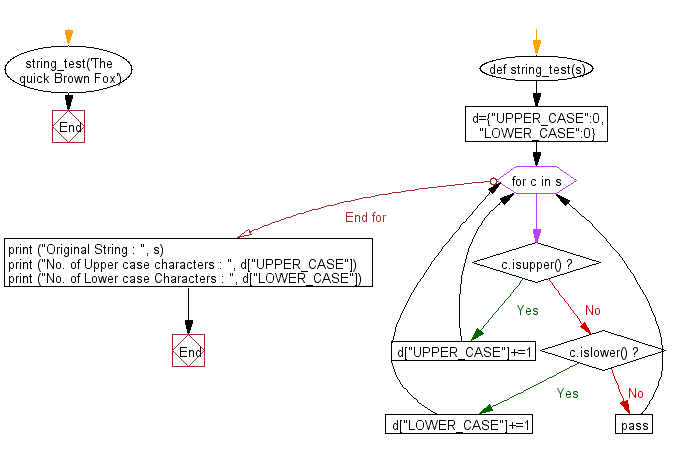
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python function to check whether a number falls in a given range.
Next: Write a Python function that takes a list and returns a new list with unique elements of the first list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics