Python Exercise: Find the sum of all the numbers in a list
Write a Python function to sum all the numbers in a list.
Sample Solution:
Python Code:
# Define a function named 'sum' that takes a list of numbers as input
def sum(numbers):
# Initialize a variable 'total' to store the sum of numbers, starting at 0
total = 0
# Iterate through each element 'x' in the 'numbers' list
for x in numbers:
# Add the current element 'x' to the 'total'
total += x
# Return the final sum stored in the 'total' variable
return total
# Print the result of calling the 'sum' function with a tuple of numbers (8, 2, 3, 0, 7)
print(sum((8, 2, 3, 0, 7)))
Sample Output:
20
Explanation:
In the exercise above the code defines a function named "sum()" that takes a list of numbers as input and returns the sum of all the numbers in the list. The final print statement demonstrates the result of calling the "sum()" function with a tuple containing the numbers (8, 2, 3, 0, 7).
Pictorial presentation:
Flowchart:
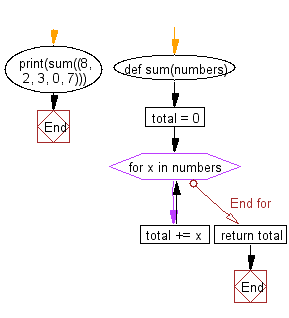
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python function to find the Max of three numbers.
Next: Write a Python function to multiply all the numbers in a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics