Python Exercise: Access a function inside a function
19. Access a Function Inside a Function
Write a Python program to access a function inside a function.
Sample Solution:
Python Code:
Sample Output:
9
Explanation:
In the exercise above the code defines nested functions and the usage of the 'nonlocal' keyword. The function "test(a)" defines a nested function "add(b)" that takes an argument 'b'. Within "add(b)", the 'nonlocal' keyword is used to modify the variable 'a' from the outer scope. The outer function "test(a)" returns the inner function "add(b)", and this returned function is assigned to the variable 'func'. Finally, it calls the function 'func' with an argument '4' and prints the result. This involves modifying the nonlocal variable 'a' and performing addition with the argument passed to 'func'.
Flowchart:
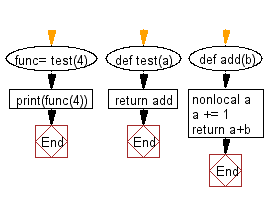
For more Practice: Solve these Related Problems:
- Write a Python program that defines an outer function containing an inner function, then returns the inner function and calls it.
- Write a Python program to implement a closure where the inner function uses a variable from the outer function's scope and returns its value.
- Write a Python program to demonstrate function nesting by creating an inner function that is conditionally executed based on an argument.
- Write a Python program to use an inner function as a callback within an outer function and print the callback’s output.
Go to:
Previous: Write a Python program to execute a string containing Python code.
Next: Write a Python program to detect the number of local variables declared in a function.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.