Python Exercise: Accept a hyphen-separated sequence of words as input and prints the sorted words
Write a Python program that accepts a hyphen-separated sequence of words as input and prints the words in a hyphen-separated sequence after sorting them alphabetically.
Sample Solution:
Python Code:
# Take user input and split it into a list based on the hyphen ("-") separator, creating a list named 'items'
items = [n for n in input().split('-')]
# Sort the elements in the 'items' list in lexicographical order (alphabetical and numerical sorting)
items.sort()
# Join the sorted elements in the 'items' list using the hyphen ("-") separator and print the resulting string
print('-'.join(items))
Sample Output:
green-red-black-white black-green-red-white
Explanation:
In the exercise above the code takes user input, splits it based on the hyphen -, and stores the parts in a list called 'items'. It then sorts these items in lexicographical order (ascending order for text strings and numerical order for numbers). Finally, it joins the sorted items using the hyphen - as a separator and prints the resulting string.
Pictorial presentation:
Flowchart:
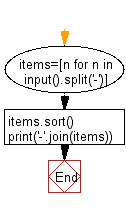
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python function to check whether a string is a pangram or not.
Next: Write a Python function to create and print a list where the values are square of numbers between 1 and 30 (both included).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics