Python Exercise: Check whether a string is a pangram or not
14. Check if a String is a Pangram
Write a Python function to check whether a string is a pangram or not.
Note : Pangrams are words or sentences containing every letter of the alphabet at least once.
For example : "The quick brown fox jumps over the lazy dog"
Sample Solution:
Python Code:
# Import the 'string' and 'sys' modules
import string
import sys
# Define a function named 'ispangram' that checks if a string is a pangram
def ispangram(str1, alphabet=string.ascii_lowercase):
# Create a set 'alphaset' containing all lowercase letters from the provided alphabet
alphaset = set(alphabet)
# Convert the input string to lowercase and create a set from it
str_set = set(str1.lower())
# Check if the set of lowercase characters in the input string covers all characters in 'alphaset'
return alphaset <= str_set
# Print the result of checking if the string is a pangram by calling the 'ispangram' function
print(ispangram('The quick brown fox jumps over the lazy dog'))
Sample Output:
True
Explanation:
In the exercise above the code defines a function named "ispangram()" that checks whether a given string is a pangram (contains all the letters of the alphabet at least once). It utilizes the 'string' module to access the lowercase alphabet and creates a set of all lowercase letters. The function then converts the input string to lowercase, creates a set from it, and checks if the set of lowercase characters in the input string covers all characters in the lowercase alphabet. Finally, it prints the result of checking if the provided string is a pangram or not.
Pictorial presentation:
Flowchart:
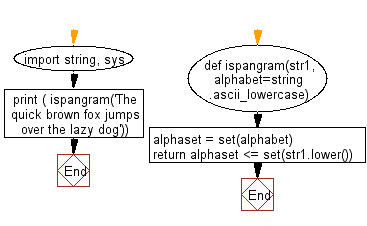
For more Practice: Solve these Related Problems:
- Write a Python function that checks if a string contains every letter of the alphabet at least once, ignoring case.
- Write a Python function that uses a set of letters to determine if a given sentence is a pangram.
- Write a Python function that removes all non-alphabet characters from a string and then checks for pangram status.
- Write a Python function that compares the set of characters in a string with the set of all lowercase letters to verify a pangram.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python function that that prints out the first n rows of Pascal's triangle.
Next: Write a Python program that accepts a hyphen-separated sequence of words as input and prints the words in a hyphen-separated sequence after sorting them alphabetically.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.