Handling UnicodeDecodeError Exception in Python file Handling program
9. Handle UnicodeDecodeError When Opening a File
Write a Python program that opens a file and handles a UnicodeDecodeError exception if there is an encoding issue.
exception UnicodeDecodeError:
Raised when a Unicode-related error occurs during decoding. It is a subclass of UnicodeError.
Content of unicode.txt:
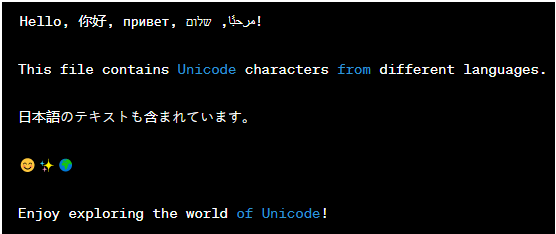
Sample Solution:
Code:
# Define a function named 'open_file' that takes 'filename' as a parameter.
def open_file(filename):
# Prompt the user to input the file encoding (ASCII, UTF-16, UTF-8) and store it in the 'encoding' variable.
encoding = input("Input the encoding (ASCII, UTF-16, UTF-8) for the file: ")
try:
# Attempt to open the file with the specified encoding in read mode and use the 'with' statement for automatic file closing.
with open(filename, 'r', encoding=encoding) as file:
# Read the contents of the file and store it in the 'contents' variable.
contents = file.read()
# Print the contents of the file.
print("File contents:")
print(contents)
except UnicodeDecodeError:
# Handle the exception if a UnicodeDecodeError occurs during file reading due to an encoding issue.
print("Error: Encoding issue occurred while reading the file.")
# Usage
# Prompt the user to input the file name and store it in the 'file_name' variable.
file_name = input("Input the file name: ")
# Call the 'open_file' function with the provided file name to open and read the file with the specified encoding.
open_file(file_name)
Explanation:
In the above exercise -
- The "open_file()" function is defined with a parameter filename, which represents the name of the file to be opened and read.
- Inside the function, the user is prompted to input the file encoding. The available options are “ASCII”, “UTF-16”, and “UTF-8”. The user's input is stored in "encoding" variable.
- The code then enters a try block, where it attempts to open the file using the specified filename and encoding using the open function with the mode set to 'r' (read mode).
- If the file is successfully opened, the file contents are read using the read method and stored in the contents variable.
- The program then prints "File contents:" to indicate that the file contents are about to be displayed.
- Finally, the file contents are printed to the console using the print function.
- If a UnicodeDecodeError exception occurs while reading the file due to an encoding issue, the code jumps to the except block. In this case, the program prints an error message indicating that an encoding issue occurred while reading the file.
- In the example usage section, the user is prompted to input the name of the file they want to open and read. The user's input is stored in the file_name variable.
- The open_file function is then called with file_name as an argument, executing the file opening and reading operation.
Output:
Input the file name: unicode.txt Input the encoding (ASCII,UTF-16,UTF-8) for the file: ASCII Error: Encoding issue occurred while reading the file.
Flowchart:
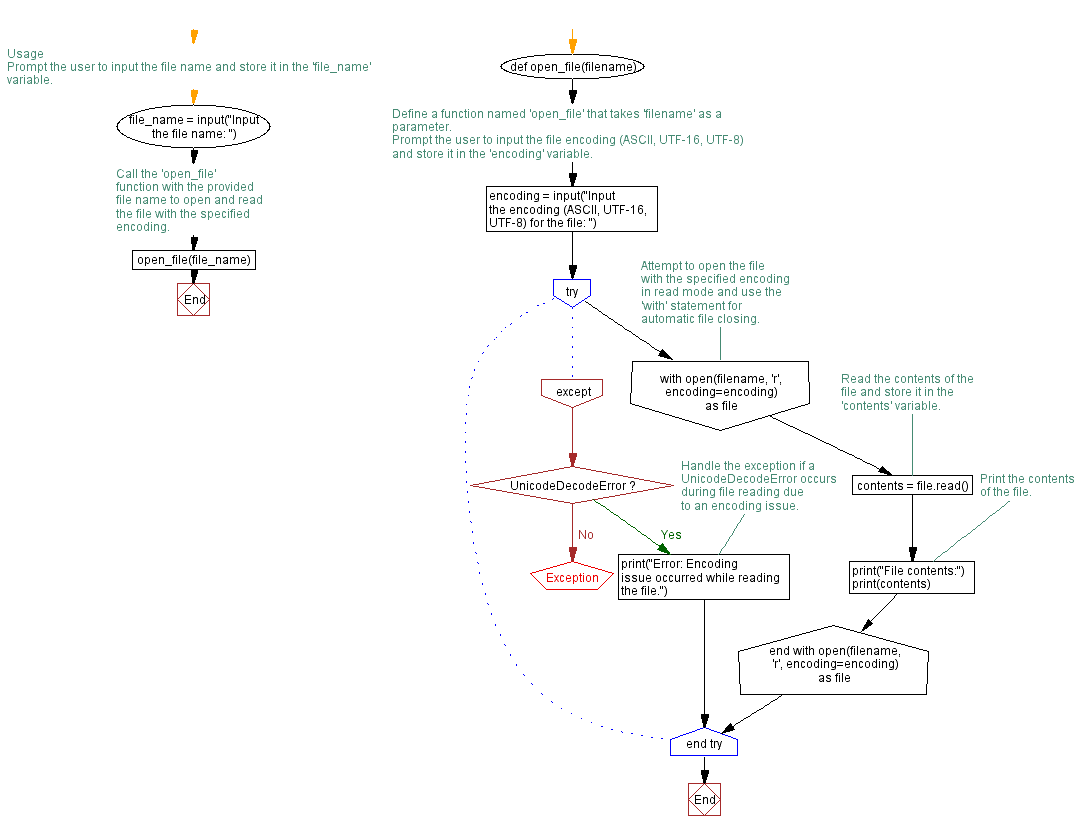
For more Practice: Solve these Related Problems:
- Write a Python program to open a file with a specific encoding and catch UnicodeDecodeError if the file contains invalid characters.
- Write a Python program to use try-except to handle UnicodeDecodeError and then attempt to reopen the file with a fallback encoding.
- Write a Python program to implement a function that reads a file and catches UnicodeDecodeError, logging the problematic line.
- Write a Python program to simulate file reading with multiple encodings and use exception handling to switch encodings on UnicodeDecodeError.
Python Code Editor:
Previous: Handling ArithmeticError exception in Python division program.
Next: Handling AttributeError Exception in Python list operation program.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.