Handling KeyboardInterrupt Exception in Python user input program
Write a Python program that prompts the user to input a number and handles a KeyboardInterrupt exception if the user cancels the input.
exception KeyboardInterrupt:
Raised when the user hits the interrupt key (normally Control-C or Delete). During execution, a check for interrupts is made regularly. The exception inherits from BaseException so as to not be accidentally caught by code that catches Exception and thus prevent the interpreter from exiting.
Sample Solution:
Code:
# Try to execute the following block of code, which may raise exceptions.
# Prompt the user to input a number and attempt to convert it to an integer, storing it in the 'n' variable.
try:
n = int(input("Input a number: "))
# If the user input is successfully converted to an integer, print the entered number.
print("You entered:", n)
# Handle the KeyboardInterrupt exception, which occurs when the user cancels the input (typically by pressing Ctrl+C).
except KeyboardInterrupt:
print("Input canceled by the user.")
Explanation:
In the above exercise,
- We use a try block to wrap the code that prompts the user to input a number. Inside the try block, we use the input function to obtain user input, and int() function to convert it to an integer.
- If the user successfully enters a number, the program proceeds to the next line, where it prints the entered number.
- However, if the user cancels the input by pressing Ctrl+C (KeyboardInterrupt), the program jumps to the except block. We catch the KeyboardInterrupt exception in the except block and print an error message informing the user that input has been canceled.
Output:
Input a number: 4 You entered: 4
Input a number: Input canceled by the user.
Flowchart:
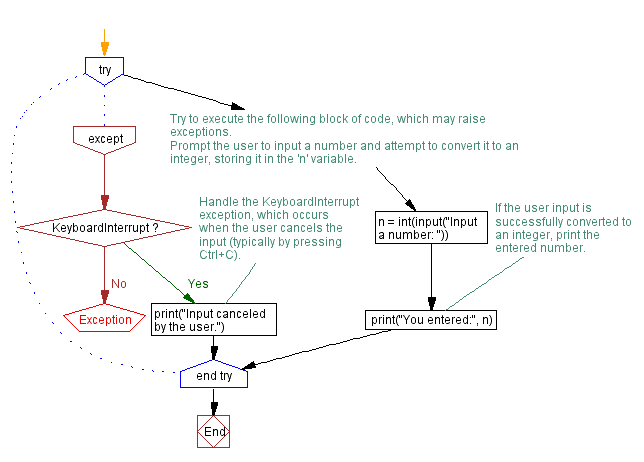
Previous: Handling IndexError Exception in Python list operation program.
Next: Handling ArithmeticError exception in Python division program.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics