Handling PermissionError Exception in Python file handling program
5. Handle PermissionError Exception When Opening a File
Write a Python program that opens a file and handles a PermissionError exception if there is a permission issue.
exception PermissionError:
Raised when trying to run an operation without the adequate access rights - for example filesystem permissions. Corresponds to errno EACCES, EPERM, and ENOTCAPABLE.
Sample Solution:
Code:
# Define a function named open_file that takes a filename as a parameter.
def open_file(filename):
try:
# Attempt to open the specified file in write mode ('w') using a 'with' statement.
with open(filename, 'w') as file:
# Try to read the contents of the file, but this code will not be executed because the file is opened in write mode.
contents = file.read()
# Print a message to indicate that the file contents will be displayed (this will not happen).
print("File contents:")
# Print the contents of the file (this will not happen).
print(contents)
except PermissionError:
# Handle the exception if there is a permission denied error while opening the file.
print("Error: Permission denied to open the file.")
# Usage
# Prompt the user to input a file name and store it in the 'file_name' variable.
file_name = input("Input a file name: ")
# Call the open_file function with the provided file name, attempting to open the file in write mode.
open_file(file_name)
Explanation:
In the above exercise,
- The "open_file()" function takes a filename parameter representing the name of the file to be opened.
- Inside the function, there is a try block that attempts to open the file using the open function in the write mode ('w'). The with statement automatically handles file closing.
- However, immediately after opening the file, it is attempted to read its contents using the read method. This is not valid because the file was opened in write mode, which does not allow reading.
- If the code reaches the except block, it means there was a PermissionError while opening the file due to a permission issue. In that case, an error message is printed indicating that permission is denied to open the file.
- In the example usage section, the user is prompted to input a file name. The "open_file()" function is then called with the provided file name as an argument.
Output:
Enter the file name: employees.csv Error: Permission denied to open the file.
Flowchart:
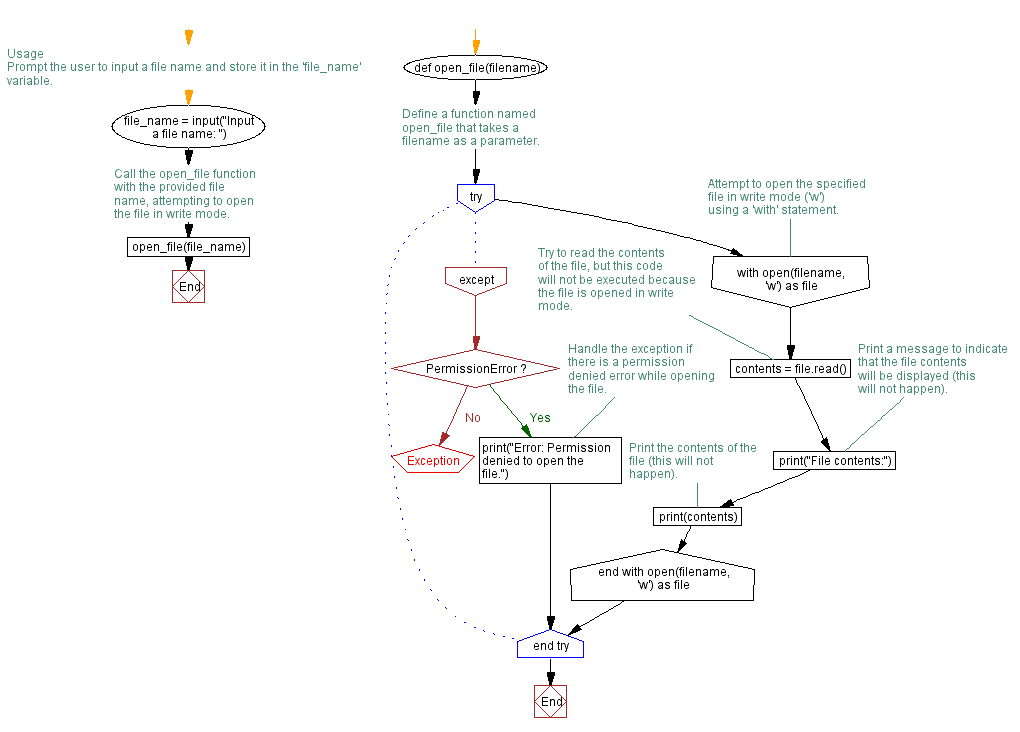
For more Practice: Solve these Related Problems:
- Write a Python program to try opening a file in write mode where permission is denied and catch the PermissionError to display an error message.
- Write a Python program to simulate file access on a read-only file and use try-except to handle PermissionError gracefully.
- Write a Python program to implement a function that attempts to open a file and catches PermissionError, prompting the user to run the script with higher privileges.
- Write a Python program to use os.chmod to change file permissions and then handle PermissionError if the operation is not permitted.
Go to:
Previous: Handling TypeError Exception in Python numeric input program.
Next: Handling IndexError Exception in Python list operation program.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.