Handling FileNotFoundError Exception in Python File Handling Program
Python Exception Handling: Exercise-3 with Solution
Write a Python program that opens a file and handles a FileNotFoundError exception if the file does not exist.
exception FileNotFoundError:
Raised when a file or directory is requested but doesn’t exist. Corresponds to errno ENOENT.
Sample Solution:
Code:
# Define a function named open_file that takes a filename as a parameter.
def open_file(filename):
try:
# Attempt to open the specified file in read mode ('r').
file = open(filename, 'r')
# Read the contents of the file and store them in the 'contents' variable.
contents = file.read()
# Print a message to indicate that the file contents will be displayed.
print("File contents:")
# Print the contents of the file.
print(contents)
# Close the file to release system resources.
file.close()
except FileNotFoundError:
# Handle the exception if the specified file is not found.
print("Error: File not found.")
# Usage
# Prompt the user to input a file name and store it in the 'file_name' variable.
file_name = input("Input a file name: ")
# Call the open_file function with the provided file name.
open_file(file_name)
Explanation:
In the above exercise, the "open_file()" function takes a "filename" parameter representing the name of the file to be opened. Inside the function, we attempt to open the file using the open function with mode 'r' to read the file.
If the file exists and can be opened successfully, we read the contents and print them. Finally, we close the file.
However, if a FileNotFoundError exception occurs because the file does not exist, the program jumps to the except block. In the except block, we handle the exception by printing an error message indicating that the file was not found.
Output:
Input a file name: test.txt Error: File not found.
Input a file name: file2.txt File contents: Raised when an operation or function receives an argument that has the right type but an inappropriate value, and the situation is not described by a more precise exception such as IndexError.
Flowchart:
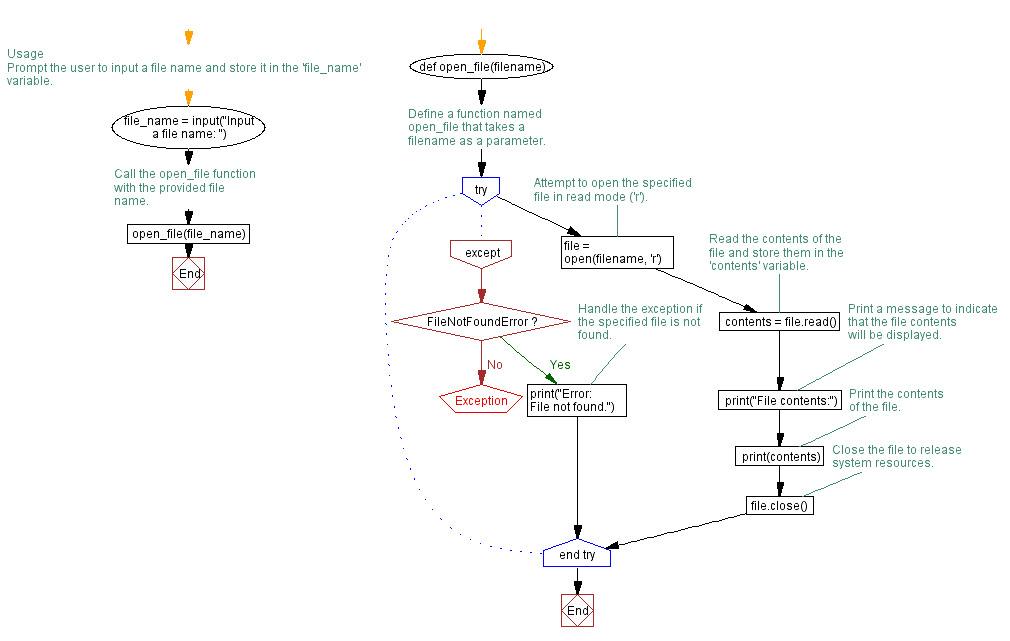
Previous: Handling ValueError Exception in Python integer input program.
Next: Handling TypeError Exception in Python numeric input program.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics