Python Exercise: Construct a specified pattern, using a nested for loop
Write a Python program to construct the following pattern, using a nested for loop.
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
Pictorial Presentation:
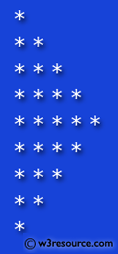
Sample Solution:
Python Code:
# Set the value of 'n' to 5 (this will determine the number of lines in the pattern)
n = 5
# Iterate through the range of numbers from 0 to 'n' (exclusive)
for i in range(n):
# Iterate through the range of numbers from 0 to 'i' (exclusive) for each 'i' in the outer loop
for j in range(i):
# Print '*' followed by a space without a new line (end="" ensures printing in the same line)
print('* ', end="")
# Move to the next line after printing '*' characters for the current 'i'
print('')
# Iterate through the range of numbers from 'n' down to 1 (inclusive), decreasing by 1 in each iteration
for i in range(n, 0, -1):
# Iterate through the range of numbers from 0 to 'i' (exclusive) for each 'i' in the outer loop
for j in range(i):
# Print '*' followed by a space without a new line (end="" ensures printing in the same line)
print('* ', end="")
# Move to the next line after printing '*' characters for the current 'i'
print('')
Sample Output:
* * * * * * * * * * * * * * * * * * * * * * * * *
Flowchart:
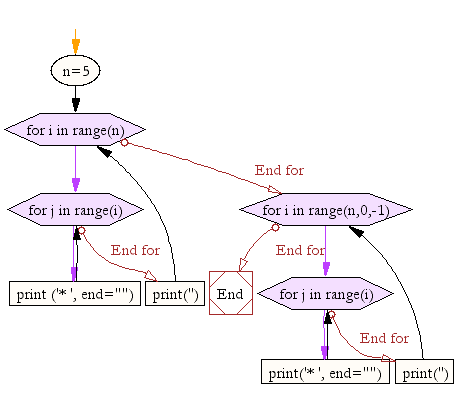
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to guess a number between 1 to 9.
Next: Write a Python program that accepts a word from the user and reverse it.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics