Python Exercise: Convert month name to a number of days
33. Month Name to Number of Days
Write a Python program to convert a month name to a number of days.
Sample Solution:
Python Code:
# Display a list of months to the user
print("List of months: January, February, March, April, May, June, July, August, September, October, November, December")
# Request input from the user to enter the name of a month and assign it to the variable 'month_name'
month_name = input("Input the name of Month: ")
# Check the input 'month_name' and provide the number of days based on the entered month
if month_name == "February":
print("No. of days: 28/29 days") # Display the number of days in February (28 or 29 days for leap years)
elif month_name in ("April", "June", "September", "November"):
print("No. of days: 30 days") # Display the number of days for months having 30 days
elif month_name in ("January", "March", "May", "July", "August", "October", "December"):
print("No. of days: 31 days") # Display the number of days for months having 31 days
else:
print("Wrong month name") # If the entered month name doesn't match any of the above conditions, display an error message
Sample Output:
List of months: January, February, March, April, May, June, July, August, September, October, November, Decemb er Input the name of Month: April No. of days: 30 days
Flowchart :
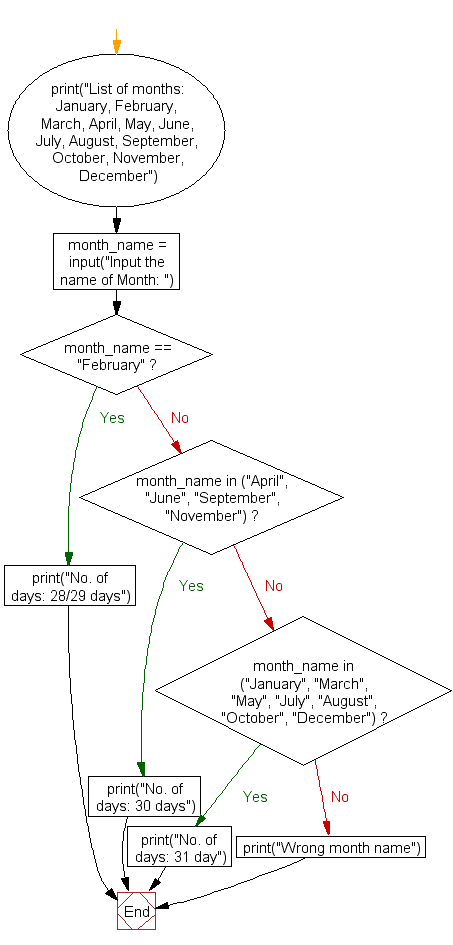
For more Practice: Solve these Related Problems:
- Write a Python program to convert a month name to its number of days, accounting for leap years in February.
- Write a Python program to use a dictionary to map month names to day counts and handle invalid input.
- Write a Python program to prompt the user for a month name and output the corresponding days using conditional checks.
- Write a Python program to implement a function that returns 28/29 for February based on a leap year flag and days for other months.
Go to:
Previous: Write a Python program to check whether an alphabet is a vowel or consonant.
Next: Write a Python program to sum of two given integers. However, if the sum is between 15 to 20 it will return 20.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.