Python Exercise: Generates a two-dimensional array
Write a Python program that takes two digits m (row) and n (column) as input and generates a two-dimensional array. The element value in the i-th row and j-th column of the array should be i*j.
Note :
i = 0,1.., m-1
j = 0,1, n-1.
Pictorial Presentation:
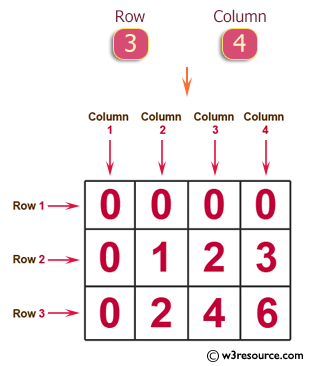
Sample Solution:
Python Code:
# Prompt the user to input the number of rows
row_num = int(input("Input number of rows: "))
# Prompt the user to input the number of columns
col_num = int(input("Input number of columns: "))
# Create a 2D list (a list of lists) filled with zeros using list comprehension
# The outer list will have 'row_num' elements and the inner lists will have 'col_num' elements
multi_list = [[0 for col in range(col_num)] for row in range(row_num)]
# Nested loop to populate the 2D list with multiplication results
for row in range(row_num):
for col in range(col_num):
# Set the value at position [row][col] in the 2D list to the product of 'row' and 'col'
multi_list[row][col] = row * col
# Print the resulting 2D list containing the multiplication table
print(multi_list)
Sample Output:
Input number of rows: 3 Input number of columns: 4 [[0, 0, 0, 0], [0, 1, 2, 3], [0, 2, 4, 6]]
Flowchart:
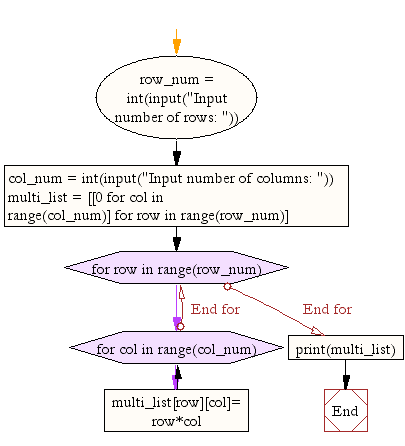
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program which iterates the integers from 1 to 50. For multiples of three print "Fizz" instead of the number and for the multiples of five print "Buzz". For numbers which are multiples of both three and five print "FizzBuzz".
Next: Write a Python program that accepts a sequence of lines (blank line to terminate) as input and prints the lines as output (all characters in lower case).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics