Python: Check whether a file path is a file or a directory
File or Directory Checker
Write a Python program to check whether a file path is a file or a directory.
Sample Solution:
Python Code:
# Import the 'os' module to access operating system functionalities.
import os
# Define the path to a file or directory named 'abc.txt'.
path = "abc.txt"
# Check if the path refers to a directory.
if os.path.isdir(path):
# Print a message indicating that it is a directory.
print("\nIt is a directory")
# Check if the path refers to a regular file.
elif os.path.isfile(path):
# Print a message indicating that it is a normal file.
print("\nIt is a normal file")
# If the path doesn't match a directory or a regular file, assume it's a special file (e.g., socket, FIFO, device file).
else:
# Print a message indicating that it is a special file.
print("It is a special file (socket, FIFO, device file)")
# Print a newline character for spacing.
print()
Sample Output:
It is a normal file
Flowchart:
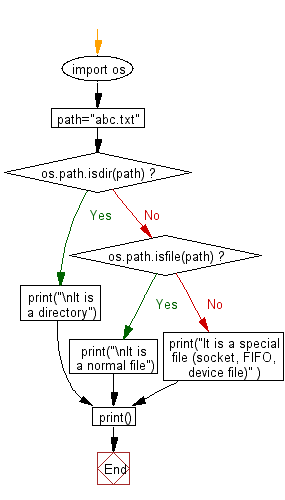
For more Practice: Solve these Related Problems:
- Write a Python program to check whether a given path exists and determine its type (file or directory).
- Write a Python program to list all files in a given directory that match a specific extension.
- Write a Python program to determine if a file is empty.
- Write a Python program to find the largest file in a directory.
Python Code Editor:
Previous: Write a Python program to count the number of occurrence of a specific character in a string.
Next: Write a Python program to get the ASCII value of a character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.