Python: Sum of the first n positive integers
Python Basic: Exercise-58 with Solution
Sum of First n Positives
Write a Python program to sum the first n positive integers.
Pictorial Presentation:
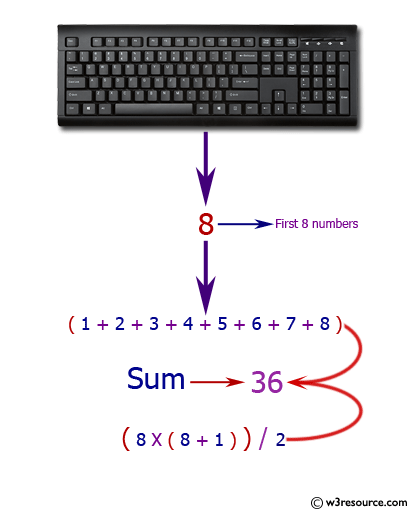
Sample Solution-1:
Python Code:
# Prompt the user for input and convert it to an integer.
n = int(input("Input a number: "))
# Calculate the sum of the first 'n' positive integers using the formula.
sum_num = (n * (n + 1)) / 2
# Print the result, indicating the sum of the first 'n' positive integers.
print("Sum of the first", n, "positive integers:", sum_num)
Sample Output:
Input a number: 2 Sum of the first 2 positive integers: 3.0
Explanation:
The input() function is used to prompt the user to input a number, and the int() function is used to convert the input to an integer value, which is stored in the variable n.
The sum of the first n positive integers is calculated using the formula sum_num = (n * (n + 1)) / 2, which is the sum of an arithmetic sequence with a first term of 1, a common difference of 1, and a total of n terms.
Finally, the print() function is used to output a message to the console in the format "Sum of the first n positive integers: sum_num", where n is the user input and sum_num is the calculated sum.
Sample Solution-2:
Python Code:
# Prompt the user for input and convert it to an integer.
n = int(input("Input an integer: "))
# Calculate the sum of the first 'n' positive integers using the built-in 'sum' function and 'range'.
result = sum(range(n+1))
# Print the result, indicating the sum of the first 'n' positive integers.
print("Sum of the first", n, "positive integers:", result)
Sample Output:
Input a number: 2 Sum of the first 2 positive integers: 3
Explanation:
In the above code -
n = int(input("Input a number: ")): Here the input() function is used to prompt the user to enter an integer, and the int() function is used to convert the input to an integer value, which is stored in the variable n.
sum_num = (n * (n + 1)) / 2: The range() function is then used to generate a sequence of integers from 0 to n inclusive, which is passed as an argument to the sum() function. The sum() function calculates the sum of the sequence and stores the result in the variable result.
print("Sum of the first", n ,"positive integers:", sum_num): Finally, the print() function is used to output a message to the console in the format "Sum of the first n positive integers: result", where n is the user input and result is the calculated sum.
Python Code Editor:
Previous: Write a program to get execution time (in seconds) for a Python method.
Next: Write a Python program to convert height (in feet and inches) to centimeters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/python-basic-exercise-58.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics