Python: Get the current username
Python Basic: Exercise-54 with Solution
Write a Python program to get the current username.
Sample Solution-1:
Python Code:
# Import the 'getpass' module to access user-related functionality, including getting the current username.
import getpass
# Use the 'getuser()' function from the 'getpass' module to retrieve the current username.
# Then, print the username to the console.
print(getpass.getuser())
Sample Output:
trinket
Explanation:
The above Python code imports the ‘getpass’ module and uses it to print the username of the current user to the console output.
The ‘getpass’ module provides a way to get the username of the current user, as well as prompt the user to enter a password without echoing the input to the console.
getpass.getuser() - This function checks the environment variables LOGNAME, USER, LNAME and USERNAME, in order, and returns the value of the first one which is set to a non-empty string. If none are set, the login name from the password database is returned on systems which support the pwd module, otherwise, an exception is raised.
The output of the code will be the username of the current user, which is retrieved from the operating system.
Flowchart:
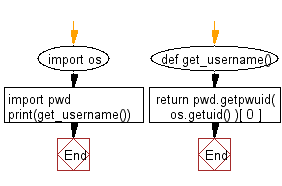
Sample Solution-2:
Python Code:
# Import the 'os' and 'pwd' modules to access operating system and user-related functionality.
import os
import pwd
# Define a function 'get_username' to retrieve the username of the current user.
def get_username():
# Use 'os.getuid()' to get the user ID of the current user, and then pass it to 'pwd.getpwuid' to get the user information.
# The '[0]' index extracts the username from the user information.
return pwd.getpwuid(os.getuid())[0]
# Call the 'get_username' function and print the result to the console.
print(get_username())
Sample Output:
trinket
Explanation:
The ‘OS’ module provides a portable way of using operating system dependent functionality.
This ‘pwd’ module provides access to the Unix user account and password database. It is available on all Unix versions.
The get_username() function defined in the code uses the os.getuid() function to get the user ID of the current process, and then passes this ID to the pwd.getpwuid() function to get a pwd.struct_passwd object representing the user account information.
The username of the user is obtained from the pwd.struct_passwd object using its pw_name attribute. The get_username() function returns this username as a string.
Finally, the print() function prints the username returned by get_username() to the console.
Flowchart:
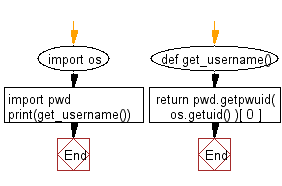
Python Code Editor:
Previous: Write a python program to access environment variables.
Next: Write a Python to find local IP addresses using Python's stdlib.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/python-basic-exercise-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics