Python: Print all even numbers from a given numbers list in the same order and stop the printing if any numbers that come after 237 in the sequence
Even Numbers Until 237
Write a Python program to print all even numbers from a given list of numbers in the same order and stop printing any after 237 in the sequence.
Sample numbers list:
numbers = [ 386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345, 399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217, 815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717, 958,743, 527 ]
Sample Solution:
Python Code:
# Define a list of numbers.
numbers = [
386, 462, 47, 418, 907, 344, 236, 375, 823, 566, 597, 978, 328, 615, 953, 345,
399, 162, 758, 219, 918, 237, 412, 566, 826, 248, 866, 950, 626, 949, 687, 217,
815, 67, 104, 58, 512, 24, 892, 894, 767, 553, 81, 379, 843, 831, 445, 742, 717,
958,743, 527
]
# Iterate through the numbers in the list.
for x in numbers:
if x == 237:
print(x) # Print the number if it's 237.
break # Exit the loop if 237 is found.
elif x % 2 == 0:
print(x) # Print the number if it's even.
Sample Output:
386 462 418 344 236 566 978 328 162 758 918 237
Explanation:
The said code defines a list called "numbers" with a set of integers. Then it uses a for loop to iterate through each element in the "numbers" list.
For each iteration, it checks if the current element is equal to 237, if true it prints the current element and exit the loop using the "break" statement.
If the current element is not equal to 237, it checks if the current element is even, if true it prints the current element.
Using this logic, the code will only print the number 237 and even numbers of the list. If 237 was not found in the list, the code will print all the even numbers in the list.
Flowchart:
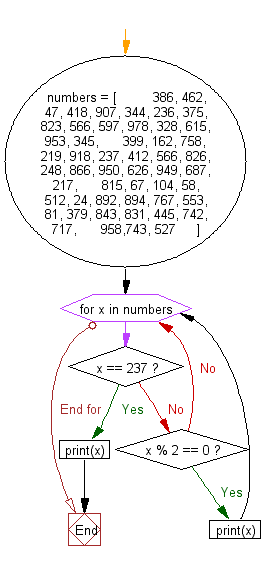
Python Code Editor:
Previous: Write a Python program to concatenate all elements in a list into a string and return it.
Next: Write a Python program to print out a set containing all the colors from a list which are not present in another list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics