Python: n (non-negative integer) copies of the first 2 characters of a given string
String Prefix Copies
Write a Python program to get n (non-negative integer) copies of the first 2 characters of a given string. Return n copies of the whole string if the length is less than 2.
Pictorial Presentation:
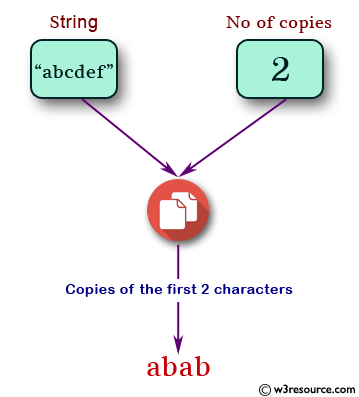
Sample Solution:
Python Code:
# Define a function called substring_copy that takes two parameters: text (a string) and n (an integer).
def substring_copy(text, n):
# Set the initial value of flen (substring length) to 2.
flen = 2
# Check if flen is greater than the length of the input text.
if flen > len(text):
# If flen is greater, set it to the length of the text to avoid going out of bounds.
flen = len(text)
# Extract a substring of length flen from the beginning of the text.
substr = text[:flen]
# Initialize an empty string result to store the concatenated substrings.
result = ""
# Iterate n times to concatenate the substring to the result.
for i in range(n):
result = result + substr
# Return the final result after concatenating substrings.
return result
# Call the substring_copy function with two different inputs and print the results.
print(substring_copy('abcdef', 2)) # Output: "abab" (substring "ab" repeated 2 times)
print(substring_copy('p', 3)) # Output: "pp" (substring "p" repeated 3 times)
Sample Output:
abab ppp
Explanation:
The said code defines a function called "substring_copy" that takes two arguments, a string "text" and an integer "n".
Inside the function, it creates a variable "flen" and assigns it the value of 2. Next, it checks whether the value of "flen" is greater than the length of the input string "text". If true it assigns the value of len(text) to "flen" otherwise it creates a variable "substr" and assigns it the value of the first two characters of the input string "text" using string slicing method.
Then it creates an empty string called "result" and uses a for loop to iterate "n" times. During each iteration it concatenates the "substr" to the "result" string. Finally, it returns the value of the "result" variable.
The last two lines of code call the function, with the input values ('abcdef', 2) and ('p', 3) and print the results. The first call will return 'abab' and the second will return 'ppp'.
Flowchart:
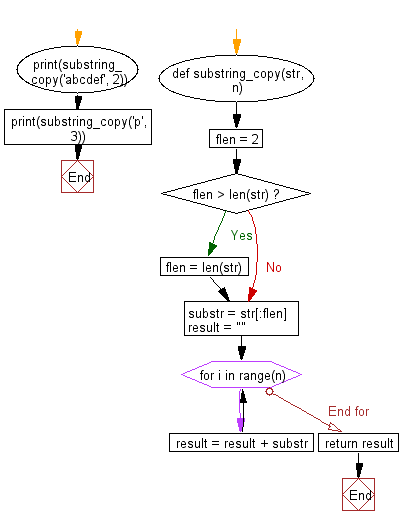
Python Code Editor:
Previous: Write a Python program to count the number 4 in a given list.
Next: Write a Python program to test whether a passed letter is a vowel or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics