Python: Get a string which is n (non-negative integer) copies of a given string
String Copy Generator
Write a Python program that returns a string that is n (non-negative integer) copies of a given string.
Pictorial Presentation:
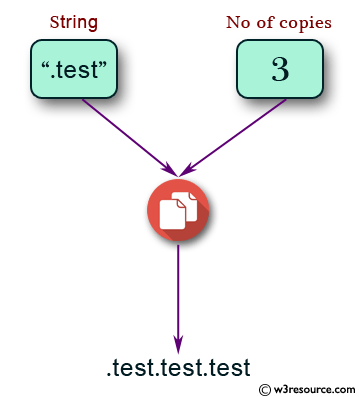
Sample Solution:
Python Code:
# Define a function named "larger_string" that takes two parameters, "text" and "n"
def larger_string(text, n):
# Initialize an empty string variable named "result"
result = ""
# Use a for loop to repeat the "text" "n" times and concatenate it to the "result"
for i in range(n):
result = result + text
# Return the final "result" string
return result
# Call the "larger_string" function with the arguments 'abc' and 2, then print the result
print(larger_string('abc', 2))
# Call the "larger_string" function with the arguments '.py' and 3, then print the result
print(larger_string('.py', 3))
Sample Output:
abcabc .py.py.py
Explanation:
The said code defines a function called "larger_string" which takes two arguments: "text" and "n". The function will concatenate the "text" argument "n" number of times and return the result as a new string.
The function starts by initializing an empty string variable called "result". Then, it uses a for loop to iterate over a range of numbers, starting from 0 and ending at "n". On each iteration, the function takes the current value of "result" and adds the "text" argument to it, effectively concatenating the "text" argument with itself "n" times.
The last two lines of code call the function, with different arguments.
In the first case the function takes 'abc' as text and 2 as the n, the function will concatenate 'abc' two times and return 'abcabc'
In the second case the function takes '.py' as text and 3 as the n, the function will concatenate '.py' three times and return '.py.py.py'
The function returns the concatenated string as the final output.
Flowchart:
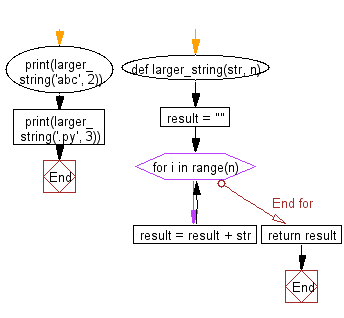
Python Code Editor:
Previous: Write a Python program to get a new string from a given string where "Is" has been added to the front. If the given string already begins with "Is" then return the string unchanged.
Next: Write a Python program to find whether a given number (accept from the user) is even or odd, print out an appropriate message to the user.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics