Python: Calculate the sum of three given numbers, if the values are equal then return thrice of their sum
Python Basic: Exercise-18 with Solution
Triple Sum Calculator
Write a Python program to calculate the sum of three given numbers. If the values are equal, return three times their sum.
Pictorial Presentation:
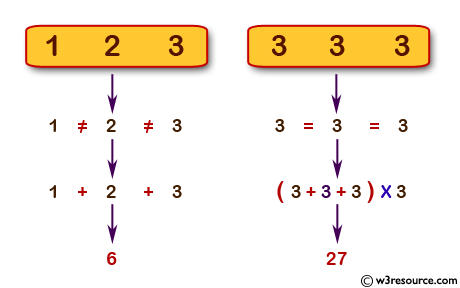
Sample Solution:
Python Code:
# Define a function named "sum_thrice" that takes three integer parameters: x, y, and z
def sum_thrice(x, y, z):
# Calculate the sum of x, y, and z
sum = x + y + z
# Check if x, y, and z are all equal (all three numbers are the same)
if x == y == z:
# If they are equal, triple the sum
sum = sum * 3
# Return the final sum
return sum
# Call the "sum_thrice" function with the arguments (1, 2, 3) and print the result
print(sum_thrice(1, 2, 3))
# Call the "sum_thrice" function with the arguments (3, 3, 3) and print the result
print(sum_thrice(3, 3, 3))
Sample Output:
6 27
Explanation:
The said script defines a function called sum_thrice(x, y, z) that takes three integers as arguments and returns their sum. The function, however, multiplies the sum by 3 if all three integers are equal.
Now the script calls the function twice with the input values (1,2,3) and (3,3,3).
Whenever 1, 2, and 3 are input values, the three inputs are not equal, so it returns 6.
Whenever 3, 3, and 3 are input values, the three inputs are equal so the function multiplies the sum by 3 and returns 27.
Depending on the inputs, the if statement holds true only if x == y == z, otherwise it holds False.
Flowchart:
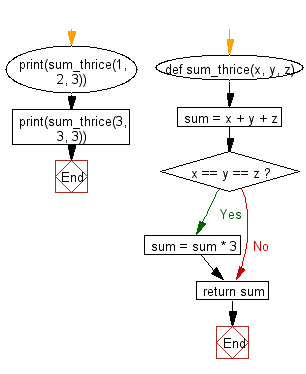
Python Code Editor:
Previous: Write a Python program to test whether a number is within 100 of 1000 or 2000.
Next: Write a Python program to get a new string from a given string where "Is" has been added to the front. If the given string already begins with "Is" then return the string unchanged.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/python-basic-exercise-18.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics