Python: Test whether a number is within 100 of 1000 or 2000
Number Range Tester
Write a Python program to test whether a number is within 100 of 1000 or 2000.
Python abs(x) function:
The function returns the absolute value of a number. The argument may be an integer or a floating point number. If the argument is a complex number, its magnitude is returned.
Sample Solution:
Python Code:
# Define a function named "near_thousand" that takes an integer parameter "n"
def near_thousand(n):
# Check if the absolute difference between 1000 and n is less than or equal to 100
# OR check if the absolute difference between 2000 and n is less than or equal to 100
return ((abs(1000 - n) <= 100) or (abs(2000 - n) <= 100))
# Call the "near_thousand" function with the argument 1000 and print the result
print(near_thousand(1000))
# Call the "near_thousand" function with the argument 900 and print the result
print(near_thousand(900))
# Call the "near_thousand" function with the argument 800 and print the result
print(near_thousand(800))
# Call the "near_thousand" function with the argument 2200 and print the result
print(near_thousand(2200))
Sample Output:
True True False False
Explanation:
The said script defines a function called near_thousand(n) that takes an integer as an argument and returns True if the input is within 100 of either 1000 or 2000, and False otherwise. The function uses the abs() function, which returns the absolute value of a number, to determine the difference between the input and 1000 or 2000.
Now the script calls the function four time with the input values of 1000, 900, 800 and 2200 respectively.
When the input value is 1000, it returns True because the difference between 1000 and 1000 is 0, which is less than 100.
When the input value is t 900, it returns True because the difference between 900 and 1000 is 100, which is less than 100.
When the input value is 800, it returns False because the difference between 800 and 1000 is 200, which is not less than or equal to 100.
When the input value is 2200, it returns False because the difference between 2200 and 2000 is 200, which is not less than or equal to 100.
Flowchart:
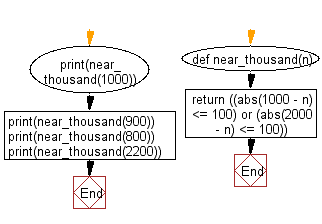
For more Practice: Solve these Related Problems:
- Write a Python program to check if a number is within 50 of 500 or 1000.
- Write a script that verifies if a number falls within multiple predefined ranges.
- Write a Python program that checks if a number is within a user-defined range.
- Write a script that finds the nearest multiple of 100 for a given number.
Go to:
Previous: Write a Python program to get the difference between a given number and 17, if the number is greater than 17 return double the absolute difference. Python Code Editor:
Next: Write a Python program to calculate the sum of three given n
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.