Python: Find the maximum and minimum numbers from a sequence of numbers
Python Basic: Exercise-148 with Solution
Write a Python function to find the maximum and minimum numbers from a sequence of numbers.
Note: Do not use built-in functions.
Sample Solution:
Python Code :
# Define a function named 'max_min' that takes a list 'data' as its argument.
def max_min(data):
# Initialize two variables 'l' and 's' with the first element of the 'data' list.
l = data[0] # 'l' is used to keep track of the maximum value.
s = data[0] # 's' is used to keep track of the minimum value.
# Iterate through each number 'num' in the 'data' list.
for num in data:
# Check if the current number 'num' is greater than the current maximum 'l'.
if num > l:
l = num # If 'num' is greater, update 'l' with 'num'.
# Check if the current number 'num' is smaller than the current minimum 's'.
elif num < s:
s = num # If 'num' is smaller, update 's' with 'num'.
# Return the maximum 'l' and minimum 's' values as a tuple.
return l, s
# Call the 'max_min' function with a list of numbers and print the result.
print(max_min([0, 10, 15, 40, -5, 42, 17, 28, 75]))
Sample Output:
(75, -5)
Pictorial Presentation:
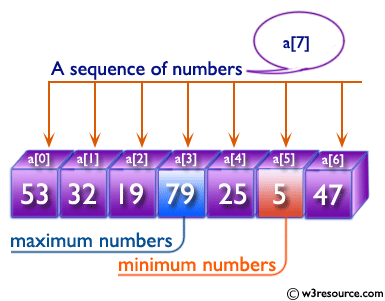
Flowchart:
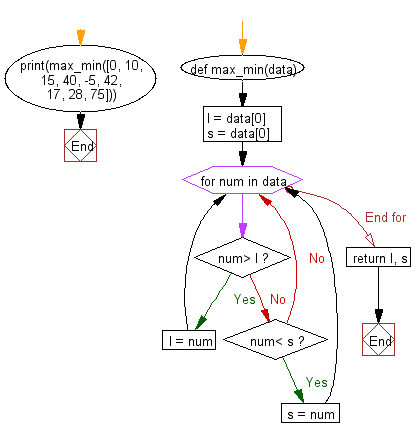
Python Code Editor :
Previous: Write a Python function to check whether a number is divisible by another number. Accept two integers values form the user.
Next: Write a Python function that takes a positive integer and returns the sum of the cube of all the positive integers smaller than the specified number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/python-basic-exercise-148.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics