Python: Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones of same length
Consecutive Zero-One Checker
Write a Python program to check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones of same length in a given string. Return True/False.
Sample Solution-1:
Python Code:
# Define a function 'test' that checks if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in a string 'str1'.
def test(str1):
# Use a 'while' loop to repeatedly find and replace '01' with an empty string in 'str1'.
while '01' in str1:
str1 = str1.replace('01', '')
# Check if the length of the modified 'str1' is equal to 0 and return the result.
return len(str1) == 0
# Define the input string 'str1'.
str1 = "01010101"
# Print the original sequence.
print("Original sequence:", str1)
# Print the result of the 'test' function for 'str1'.
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
# Repeat the same process for other test cases.
str1 = "00"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "000111000111"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "00011100011"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
Sample Output:
Original sequence: 01010101 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: True Original sequence: 00 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False Original sequence: 000111000111 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: True Original sequence: 00011100011 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False
Flowchart:
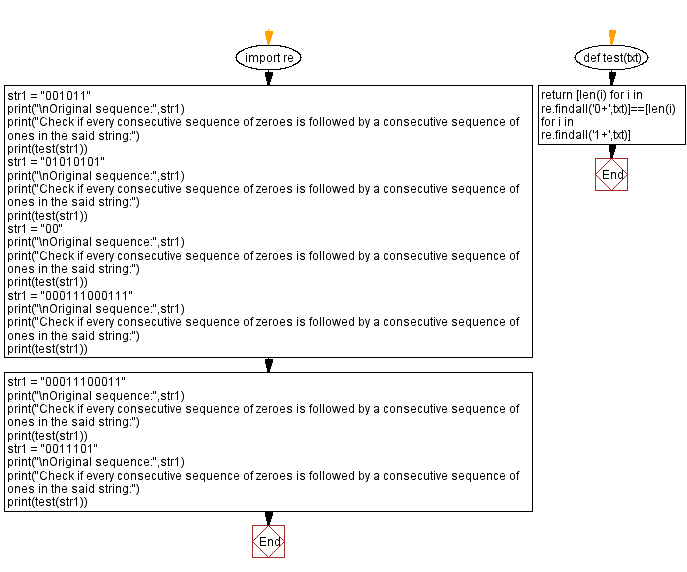
Sample Solution-2:
Python Code:
# Define a function 'test' that checks if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in a string 'str1'.
def test(str1):
# Initialize an empty list 'temp' to store '0's.
temp = []
# Iterate through each character 'x' in the input string 'str1'.
for x in str1:
# If 'x' is '0', append '0' to the 'temp' list.
if x == '0':
temp.append('0')
else:
# If 'x' is not '0', remove the last element from 'temp'.
temp.pop()
# Check if 'temp' is empty (all '0's were matched by '1's) and return the result.
return not temp
# Define the input string 'str1'.
str1 = "01010101"
# Print the original sequence.
print("Original sequence:", str1)
# Print the result of the 'test' function for 'str1'.
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
# Repeat the same process for other test cases.
str1 = "00"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "000111000111"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "00011100011"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
Sample Output:
Original sequence: 01010101 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: True Original sequence: 00 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False Original sequence: 000111000111 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: True Original sequence: 00011100011 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False
Flowchart:
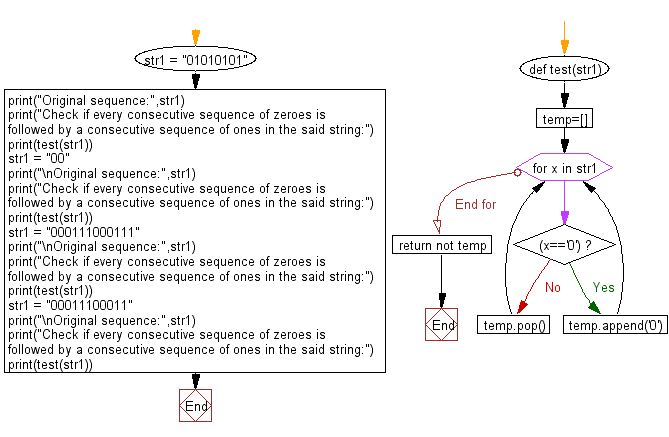
Python Code Editor:
Previous: Write a python program to convert decimal to hexadecimal.
Next: Write a Python program to make a request to a web page, and test the status code, also display the html code of the specified web page.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics