Python: Remove the first item from a specified list
Remove First List Item
Write a Python program to remove the first item from a specified list.
Sample Solution-1:
Python Code:
# Create a list of color names
color = ["Red", "Black", "Green", "White", "Orange"]
# Print the original list elements
print("Original list elements:")
print(color)
# Remove the first element (element at index 0) from the list
del color[0]
# Print the list after removing the first color
print("After removing the first color: ")
print(color)
print()
Sample Output:
Original list elements: ['Red', 'Black', 'Green', 'White', 'Orange'] After removing the first color: ['Black', 'Green', 'White', 'Orange']
Sample Solution-2:
Python Code:
# Create a list of color names
color = ["Red", "Black", "Green", "White", "Orange"]
# Print the original list elements
print("Original list elements:")
print(color)
# Print a message indicating the operation that will be performed
print("\nAfter removing the first element from the said list:")
# Create a new list 'new_color' by slicing the original list from the second element (index 1) to the end
new_color = color[1:]
# Print the modified list after removing the first element
print(new_color)
Sample Output:
Original list elements: ['Red', 'Black', 'Green', 'White', 'Orange'] After removing the first element from the said list: ['Black', 'Green', 'White', 'Orange']
Sample Solution-3:
Python Code:
# Create a list of color names
color = ["Red", "Black", "Green", "White", "Orange"]
# Print a message indicating the original list elements
print("Original list elements:")
# Print the original list elements
print(color)
# Print a message indicating the operation that will be performed
print("\nAfter removing the first element from the said list:")
# Remove the element "Red" from the list
color.remove("Red")
# Print the modified list after removing the element
print(color)
Sample Output:
Original list elements: ['Red', 'Black', 'Green', 'White', 'Orange'] After removing the first element from the said list: ['Black', 'Green', 'White', 'Orange']
Sample Solution-4:
- Use slice notation to return the last element if the list's length is more than 1.
- Otherwise, return the whole list.
Python Code:
# Define a function named "tail" that takes a list "lst" as input.
def tail(lst):
# Check if the length of the list is greater than 1.
if len(lst) > 1:
# If the list has more than one element, return a new list containing all elements except the first one.
return lst[1:]
else:
# If the list has only one element or is empty, return the original list.
return lst
# Call the "tail" function with different lists and print the results.
print(tail([1, 2, 3, 4])) # Should print [2, 3, 4]
print(tail([1])) # Should print [1] (no change)
print(tail(["Red", "Black", "Green", "White", "Orange"])) # Should print ["Black", "Green", "White", "Orange"]
Sample Output:
[2, 3, 4] [1] ['Black', 'Green', 'White', 'Orange']
Flowchart:
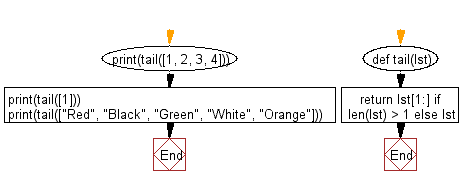
Python Code Editor:
Previous: Write a Python program to make file lists from current directory using a wildcard.
Next: Write a Python program to input a number, if it is not a number generates an error message.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics