Python: Access and print a URL's content to the console
URL Content Printer
Write a Python program to access and print a URL's content to the console.
Sample Solution-1:
Python Code:
# Import the HTTPConnection class from the http.client module.
from http.client import HTTPConnection
# Create an HTTPConnection object for the "example.com" host.
conn = HTTPConnection("example.com")
# Send a GET request to the root path ("/") of the host.
conn.request("GET", "/")
# Get the response from the server.
result = conn.getresponse()
# Retrieve the entire contents of the response.
contents = result.read()
# Print the contents of the response.
print(contents)
Sample Output:
b'<!doctype html>\n<html>\n<head>\n <title>Example Domain</title >\n\n <meta charset="utf-8" />\n <meta http-equiv="Content-ty pe" content="text/html; charset=utf-8" />\n <meta name="viewport " content="width=device-width, initial-scale=1.0" />\n <style type ="text/css">\n body {\n background-color: #f0f0f2;\n margin: 0;\n padding: 0;\n font-family: "Open Sans ", "Helvetica Neue", Helvetica, Arial, sans-serif;\n \n } \n div {\n width: 600px;\n margin: 5em auto;\n padding: 50px;\n background-color: #fff;\n border -radius: 1em;\n }\n a:link, a:visited {\n color: #3848 8f;\n text-decoration: none;\n }\n @media (max-width: 700px) {\n body {\n background-color: #fff;\n }\n div {\n width: auto;\n margin: 0 auto;\n border-radius: 0;\n padding: 1em;\ n }\n }\n </style> \n</head>\n\n<body>\n<div>\n <h1>Example Domain</h1>\n <p>This domain is established to be us ed for illustrative examples in documents. You may use this\n do main in examples without prior coordination or asking for permissio n.</p>\n <p><a href="http://www.iana.org/domains/example">More i nformation...</a></p>\n</div>\n</body>\n</html>\n'
Flowchart:
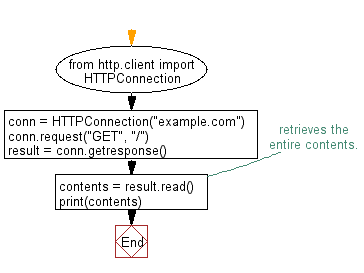
Sample Solution-2:
Python Code:
# Import the requests library to make HTTP requests.
import requests
# Send an HTTP GET request to the 'https://google.com/' URL and store the response.
data = requests.get('https://google.com/')
# Access the text content of the response, which contains the webpage's HTML.
webpage_text = data.text
# Print the HTML content of the webpage.
print(webpage_text)
Sample Output:
<!doctype html><html itemscope="" itemtype="http://schema.org/WebPage" lang="en"><head><meta content="Search the world's information, including webpages, images, videos and more. Google has many special features to help you find exactly what you're looking for." name="description"><meta content="noodp" name="robots"><meta content="text/html; charset=UTF-8" http-equiv="Content-Type"><meta content="/images/branding/googleg/1x/googleg_standard_color_128dp.png" itemprop="image"><title>Google</title><script nonce="SipCO1RV/67CawSQ8sCuFw==">(function(){window.google={kEI:'h9u1YNW3GsOVwbkPnOaBkA4',kEXPI:'0,772215,1,6543,523777,56873,954,756,4348,207,4804,2316,383,246,5,1354,4936,314,6386,1116130,1197734,518,30,328985,51224,16114,28683,1121,16452,4859,1361,9290,3023,17586,4020,978,13228,2054,1793,4192,6430,1142,6290,7095,4521,2774,921,5079,889,704,1279,1042,1170,530,149,1103,840,517,1466,4314,108,3406,606,2023,1777,520,2677,11993,3227,2845,7,5599,6755,5096,7876,4929,108,1483,1924,908,2,941,5011,10313,432,3,1590,1,820,1,4623,149,5990,5333,991,1661,4,1528,2304,1236,5227,576,74,1717,266,2627,2014,4067,7434,3824,3050,2658,4242,519,912,1684,30,3854,9774,2305,638,37,1457,5586,3772,5993,770,665,2145,3673,2539,4094,3138,6,908,3,3541,1,4174,1175,4596,4317,454,1,1807,283,38,874,60,5934,1258,5494,432,552,4608,180,2,1394,756,658,1,87,1,22,1281,1715,2,3035,22,2015,3246,894,12,397,218,4,32,4,533,1704,2,2524,110,514,4,90,530,2,61,442,185,157,254,56,4,434,350,2,1102,1016,30,520,1780,115,1160,1262,78,120,2656,56,406,2095,158,288,87,3,1,1065,172,392,3089,106,285,134,515,579,111,708,675,163,997,474,2022,29,1609,552,1,3,430,1790,1088,142,92,2,186,2000,1,233,298,267,933,1394,3,1038,920,2,108,3,35,145,2,103,235,204,3,75,52,2286,113,1711,468,387,70,319,694,178,1,305,275,321,1999,67,349,27,345,343,5638671,183,43,63,155,45,5996306,519,46,2800651,882,444,1,2,80,1,1796,1,9,2,2551,1,748,141,795,563,1,4265,1,1,2,1331,3299,843,1,2608,155,17,13,72,139,4,2,20,2,169,13,19,38,8,5,39,96,284,4,4,4,4,4,4,8,4,4,4,24,196,29,2,2,1,2,1,2,2,7,4,1,2,2,2,2,2,2,673,52,1,18,14,3,8,50,23955410,4010273,268,1678,25122,1502,2,864,1510,3,467,3,1823',kBL:'ckvK'};google.sn='webhp';google.kHL='en';})();(function(){ var f,h=[];function k(a){for(var b;a&&(!a.getAttribute||!(b=a.getAttribute("eid")));)a=a.parentNode;return b||f}function l(a){for(var b=null;a&&(!a.getAttribute||!(b=a.getAttribute("leid")));)a=a.parentNode;return b} function m(a,b,c,d,g){var e="";c||-1!=b.search("&ei=")||(e="&ei="+k(d),-1==b.search("&lei=")&&(d=l(d))&&(e+="&lei="+d));d="";!c&&window._cshid&&-1==b.search("&cshid=")&&"slh"!=a&&(d="&cshid="+window._cshid);c=c||"/"+(g||"gen_204")+"?atyp=i&ct="+a+"&cad="+b+e+"&zx="+Date.now()+d;/^http:/i.test(c)&&"https:"==window.location.protocol&&(google.ml&&google.ml(Error("a"),!1,{src:c,glmm:1}),c="");return c};f=google.kEI;google.getEI=k;google.getLEI=l;google.ml=function(){return null};google.log=function(a,b,c,d,g){if(c=m(a,b,c,d,g)){a=new Image;var e=h.length;h[e]=a;a.onerror=a.onload=a.onabort=function(){delete h[e]};a.src=c}};google.logUrl=m;}).call(this);(function(){ google.y={};google.sy=[];google.x=function(a,b){if(a)var c=a.id;else{do c=Math.random();while(google.y[c])}google.y[c]=[a,b];return!1};google.sx=function(a){google.sy.push(a)};google.lm=[];google.plm=function(a){google.lm.push.apply(google.lm,a)};google.lq=[];google.load=function(a,b,c){google.lq.push([[a],b,c])};google.loadAll=function(a,b){google.lq.push([a,b])};google.bx=!1;google.lx=function(){};}).call(this);google.f={};(function(){ document.documentElement.addEventListener("submit",function(b){var a;if(a=b.target){var c=a.getAttribute("data-submitfalse");a="1"==c||"q"==c&&!a.elements.q.value?!0:!1}else a=!1;a&&(b.preventDefault(),b.stopPropagation())},!0);document.documentElement.addEventListener("click",function(b){var a;a:{for(a=b.target;a&&a!=document.documentElement;a=a.parentElement)if("A"==a.tagName){a="1"==a.getAttribute("data-nohref");break a}a=!1}a&&b.preventDefault()},!0);}).call(this);</script><style>#gbar,#guser{font-size:13px;padding-top:1px !important;}#gbar{height:22px}#guser{padding-bottom:7px !important;text-align:right}.gbh,.gbd{border-top:1px solid #c9d7f1;font-size:1px}.gbh{height:0;position:absolute;top:24px;width:100%}@media all{.gb1{height:22px;margin-right:.5em;vertical-align:top}#gbar{float:left}}a.gb1,a.gb4{text-decoration:underline !important}a.gb1,a.gb4{color:#00c !important}.gbi .gb4{color:#dd8e27 !important}.gbf .gb4{color:#900 !important} </style><style>body,td,a,p,.h{font-family:arial,sans-serif}body{margin:0;overflow-y:scroll}#gog{padding:3px 8px 0}td{line-height:.8em}.gac_m td{line-height:17px}form{margin-bottom:20px}.h{color:#1558d6}em{font-weight:bold;font-style:normal}.lst{height:25px;width:496px}.gsfi,.lst{font:18px arial,sans-serif}.gsfs{font:17px arial,sans-serif}.ds{display:inline-box;display:inline-block;margin:3px 0 4px;margin-left:4px}input{font-family:inherit}body{background:#fff;color:#000}a{color:#4b11a8;text-decoration:none}a:hover,a:active{text-decoration:underline}.fl a{color:#1558d6}a:visited{color:#4b11a8}.sblc{padding-top:5px}.sblc a{display:block;margin:2px 0;margin-left:13px;font-size:11px}.lsbb{background:#f8f9fa;border:solid 1px;border-color:#dadce0 #70757a #70757a #dadce0;height:30px}.lsbb{display:block}#WqQANb a{display:inline-block;margin:0 12px}.lsb{background:url(/images/nav_logo229.png) 0 -261px repeat-x;border:none;color:#000;cursor:pointer;height:30px;margin:0;outline:0;font:15px arial,sans-serif;vertical-align:top}.lsb:active{background:#dadce0}.lst:focus{outline:none}</style><script nonce="SipCO1RV/67CawSQ8sCuFw=="></script></head><body bgcolor="#fff"><script nonce="SipCO1RV/67CawSQ8sCuFw==">(function(){var src='/images/nav_logo229.png';var iesg=false;document.body.onload = function(){window.n && window.n();if (document.images){new Image().src=src;} if (!iesg){document.f&&document.f.q.focus();document.gbqf&&document.gbqf.q.focus();} } })();</script><div id="mngb"><div id=gbar><nobr><b class=gb1>Search</b> <a class=gb1 href="https://www.google.com/imghp?hl=en&tab=wi">Images</a> <a class=gb1 href="https://maps.google.com/maps?hl=en&tab=wl">Maps</a> <a class=gb1 href="https://play.google.com/?hl=en&tab=w8">Play</a> <a class=gb1 href="https://www.youtube.com/?gl=US&tab=w1">YouTube</a> <a class=gb1 href="https://news.google.com/?tab=wn">News</a> <a class=gb1 href="https://mail.google.com/mail/?tab=wm">Gmail</a> <a class=gb1 href="https://drive.google.com/?tab=wo">Drive</a> <a class=gb1 style="text-decoration:none" href="https://www.google.com/intl/en/about/products?tab=wh"><u>More</u> »</a></nobr></div><div id=guser width=100%><nobr><span id=gbn class=gbi></span><span id=gbf class=gbf></span><span id=gbe></span><a href="http://www.google.com/history/optout?hl=en" class=gb4>Web History</a> | <a href="/preferences?hl=en" class=gb4>Settings</a> | <a target=_top id=gb_70 href="https://accounts.google.com/ServiceLogin?hl=en&passive=true&continue=https://www.google.com/&ec=GAZAAQ" class=gb4>Sign in</a></nobr></div><div class=gbh style=left:0></div><div class=gbh style=right:0></div></div><center><br clear="all" id="lgpd"><div id="lga"><img alt="Google" height="92" src="/images/branding/googlelogo/1x/googlelogo_white_background_color_272x92dp.png" style="padding:28px 0 14px" width="272" id="hplogo"><br><br></div><form action="/search" name="f"><table cellpadding="0" cellspacing="0"><tr valign="top"><td width="25%"> </td><td align="center" nowrap=""><input name="ie" value="ISO-8859-1" type="hidden"><input value="en" name="hl" type="hidden"><input name="source" type="hidden" value="hp"><input name="biw" type="hidden"><input name="bih" type="hidden"><div class="ds" style="height:32px;margin:4px 0"><input class="lst" style="margin:0;padding:5px 8px 0 6px;vertical-align:top;color:#000" autocomplete="off" value="" title="Google Search" maxlength="2048" name="q" size="57"></div><br style="line-height:0"><span class="ds"><span class="lsbb"><input class="lsb" value="Google Search" name="btnG" type="submit"></span></span><span class="ds"><span class="lsbb"><input class="lsb" id="tsuid1" value="I'm Feeling Lucky" name="btnI" type="submit"><script nonce="SipCO1RV/67CawSQ8sCuFw==">(function(){var id='tsuid1';document.getElementById(id).onclick = function(){if (this.form.q.value){this.checked = 1;if (this.form.iflsig)this.form.iflsig.disabled = false;} else top.location='/doodles/';};})();</script><input value="AINFCbYAAAAAYLXpl8FlgjokBb32Dbg3kecNqk7aWrD-" name="iflsig" type="hidden"></span></span></td><td class="fl sblc" align="left" nowrap="" width="25%"><a href="/advanced_search?hl=en&authuser=0">Advanced search</a></td></tr></table><input id="gbv" name="gbv" type="hidden" value="1"><script nonce="SipCO1RV/67CawSQ8sCuFw==">(function(){ var a,b="1";if(document&&document.getElementById)if("undefined"!=typeof XMLHttpRequest)b="2";else if("undefined"!=typeof ActiveXObject){var c,d,e=["MSXML2.XMLHTTP.6.0","MSXML2.XMLHTTP.3.0","MSXML2.XMLHTTP","Microsoft.XMLHTTP"];for(c=0;d=e[c++];)try{new ActiveXObject(d),b="2"}catch(h){}}a=b;if("2"==a&&-1==location.search.indexOf("&gbv=2")){var f=google.gbvu,g=document.getElementById("gbv");g&&(g.value=a);f&&window.setTimeout(function(){location.href=f},0)};}).call(this);</script></form><div id="gac_scont"></div><div style="font-size:83%;min-height:3.5em"><br><div id="prm"><style>.szppmdbYutt__middle-slot-promo{font-size:small;margin-bottom:32px}.szppmdbYutt__middle-slot-promo a.ZIeIlb{display:inline-block;text-decoration:none}.szppmdbYutt__middle-slot-promo img{border:none;margin-right:5px;vertical-align:middle}</style><div class="szppmdbYutt__middle-slot-promo" data-ved="0ahUKEwiVl_3n7vXwAhXDSjABHRxzAOIQnIcBCAQ"><a class="ZIeIlb" href="https://www.google.com/url?q=https://www.google.com/search%3Fq%3DMemorial%2BDay&source=hpp&id=19024517&ct=4&usg=AFQjCNFT7cUhhFZa9Ubr-ZOAc5PbhHnsdA&sa=X&ved=0ahUKEwiVl_3n7vXwAhXDSjABHRxzAOIQ8IcBCAU"><img alt="A moment of remembrance on Memorial Day" height="60" src="https://www.google.com/images/hpp/us-flag.png" title="A moment of remembrance on Memorial Day" width="68"></a></div></div></div><span id="footer"><div style="font-size:10pt"><div style="margin:19px auto;text-align:center" id="WqQANb"><a href="/intl/en/ads/">Advertising Programs</a><a href="/services/">Business Solutions</a><a href="/intl/en/about.html">About Google</a></div></div><p style="font-size:8pt;color:#70757a">© 2021 - <a href="/intl/en/policies/privacy/">Privacy</a> - <a href="/intl/en/policies/terms/">Terms</a></p></span></center><script nonce="SipCO1RV/67CawSQ8sCuFw==">(function(){window.google.cdo={height:0,width:0};(function(){ var a=window.innerWidth,b=window.innerHeight;if(!a||!b){var c=window.document,d="CSS1Compat"==c.compatMode?c.documentElement:c.body;a=d.clientWidth;b=d.clientHeight}a&&b&&(a!=google.cdo.width||b!=google.cdo.height)&&google.log("","","/client_204?&atyp=i&biw="+a+"&bih="+b+"&ei="+google.kEI);}).call(this);})();</script> <script nonce="SipCO1RV/67CawSQ8sCuFw==">(function(){google.xjs={ck:'',cs:'',excm:[],pml:false};})();</script> <script nonce="SipCO1RV/67CawSQ8sCuFw==">(function(){var u='/xjs/_/js/k\x3dxjs.hp.en_US.fHmHfL28PcU.O/m\x3dsb_he,d/am\x3dAHgCLA/d\x3d1/ed\x3d1/rs\x3dACT90oFiDOwIi-4CQMcgq1j587Iy9c22hA'; var e=this||self,f=function(a){return a};var g;var l=function(a,b){this.g=b===h?a:""};l.prototype.toString=function(){return this.g+""};var h={};function m(){var a=u;google.lx=function(){n(a);google.lx=function(){}};google.bx||google.lx()} function n(a){google.timers&&google.timers.load&&google.tick&&google.tick("load","xjsls");var b=document;var c="SCRIPT";"application/xhtml+xml"===b.contentType&&(c=c.toLowerCase());c=b.createElement(c);if(void 0===g){b=null;var k=e.trustedTypes;if(k&&k.createPolicy){try{b=k.createPolicy("goog#html",{createHTML:f,createScript:f,createScriptURL:f})}catch(p){e.console&&e.console.error(p.message)}g=b}else g=b}a=(b=g)?b.createScriptURL(a):a;a=new l(a,h);c.src=a instanceof l&&a.constructor===l?a.g:"type_error:TrustedResourceUrl";var d;a=(c.ownerDocument&&c.ownerDocument.defaultView||window).document;(d=(a=null===(d=a.querySelector)||void 0===d?void 0:d.call(a,"script[nonce]"))?a.nonce||a.getAttribute("nonce")||"":"")&&c.setAttribute("nonce",d);document.body.appendChild(c);google.psa=!0};setTimeout(function(){m()},0);})();(function(){window.google.xjsu='/xjs/_/js/k\x3dxjs.hp.en_US.fHmHfL28PcU.O/m\x3dsb_he,d/am\x3dAHgCLA/d\x3d1/ed\x3d1/rs\x3dACT90oFiDOwIi-4CQMcgq1j587Iy9c22hA';})();function _DumpException(e){throw e;} function _F_installCss(c){} (function(){google.jl={blt:'none',dw:false,emtn:0,ine:false,lls:'default',pdt:0,snet:true,uwp:true};})();(function(){var pmc='{\x22d\x22:{},\x22sb_he\x22:{\x22agen\x22:true,\x22cgen\x22:true,\x22client\x22:\x22heirloom-hp\x22,\x22dh\x22:true,\x22dhqt\x22:true,\x22ds\x22:\x22\x22,\x22ffql\x22:\x22en\x22,\x22fl\x22:true,\x22host\x22:\x22google.com\x22,\x22isbh\x22:28,\x22jsonp\x22:true,\x22msgs\x22:{\x22cibl\x22:\x22Clear Search\x22,\x22dym\x22:\x22Did you mean:\x22,\x22lcky\x22:\x22I\\u0026#39;m Feeling Lucky\x22,\x22lml\x22:\x22Learn more\x22,\x22oskt\x22:\x22Input tools\x22,\x22psrc\x22:\x22This search was removed from your \\u003Ca href\x3d\\\x22/history\\\x22\\u003EWeb History\\u003C/a\\u003E\x22,\x22psrl\x22:\x22Remove\x22,\x22sbit\x22:\x22Search by image\x22,\x22srch\x22:\x22Google Search\x22},\x22nrft\x22:false,\x22ovr\x22:{},\x22pq\x22:\x22\x22,\x22refpd\x22:true,\x22rfs\x22:[],\x22sbas\x22:\x220 3px 8px 0 rgba(0,0,0,0.2),0 0 0 1px rgba(0,0,0,0.08)\x22,\x22sbpl\x22:16,\x22sbpr\x22:16,\x22scd\x22:10,\x22stok\x22:\x22IZJZWD46ZSkvf0ROksFAB2UfyP0\x22,\x22uhde\x22:false}}';google.pmc=JSON.parse(pmc);})();</script> </body></html>
Python Code Editor:
Previous: Write a Python program to get the name of the host on which the routine is running.
Next: Write a Python program to get system command output.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics