Python: Input an integer (n) and computes the value of n+nn+nnn
Python Basic: Exercise-10 with Solution
Write a Python program that accepts an integer (n) and computes the value of n+nn+nnn.
Sample value of n is 5
Python int(x, base=10):
The function returns an integer object constructed from a number or string x, or return 0 if no arguments are given. If x is a number, return x.__int__(). For floating point numbers, this truncates towards zero.
- If x is not a number or if base is given, then x must be a string, bytes, or bytearray instance representing an integer literal in radix base
- The literal can be preceded by + or - (with no space in between) and surrounded by whitespace
- A base-n literal consists of the digits 0 to n-1, with a to z (or A to Z) having values 10 to 35. The default base is 10.
- The allowed values are 0 and 2-36. Base-2, -8, and -16 literals can be optionally prefixed with 0b/0B, 0o/0O, or 0x/0X, as with integer literals in code.
Pictorial Presentation:
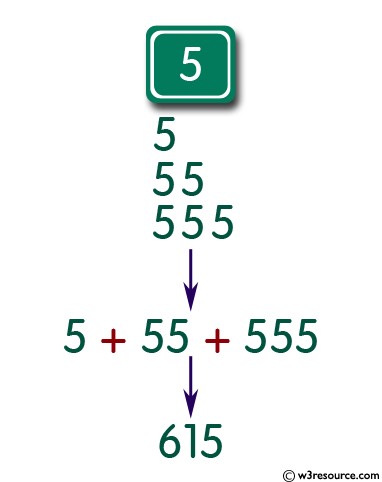
Sample Solution:
Python Code:
# Prompt the user to input an integer and store it in the variable 'a'
a = int(input("Input an integer: "))
# Create new integers 'n1', 'n2', and 'n3' by concatenating 'a' with itself one, two, and three times, respectively
n1 = int("%s" % a) # Convert 'a' to an integer
n2 = int("%s%s" % (a, a)) # Concatenate 'a' with itself and convert to an integer
n3 = int("%s%s%s" % (a, a, a)) # Concatenate 'a' with itself twice and convert to an integer
# Calculate the sum of 'n1', 'n2', and 'n3' and print the result
print(n1 + n2 + n3)
Sample Output:
615
Explanation:
The said code prompts the user to input an integer, which is then stored in the variable "a".
There after the first variable n1 is created by converting the input integer "a" to a string and then back to an integer.
The second variable n2 is created by concatenating two copies of the input integer "a" as a string and then converting that string to an integer.
The third variable n3 is created by concatenating three copies of the input integer "a" as a string and then converting that string to an integer.
Finally, the code sums the three variables n1, n2 and n3 and print the result.
Flowchart:
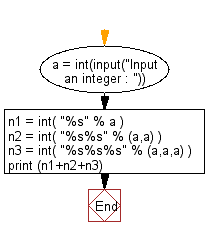
Python Code Editor:
Previous: Write a Python program to display the examination schedule. (extract the date from exam_st_date).
Next: Write a Python program to print the documents (syntax, description etc.) of Python built-in function(s).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/python-basic-exercise-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics