Python PyQt program - Temperature converter
Write a Python program that implements a temperature converter app that lets users choose between Celsius and Fahrenheit using PyQt. Customize the widget appearance based on the selected unit.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QWidget: The QWidget class is the base class of all user interface objects.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QLabel Class: The QLabel widget provides a text or image display.
QLineEdit Class: A line edit allows the user to enter and edit a single line of plain text with a useful collection of editing functions, including undo and redo, cut and paste, and drag and drop.
QPushButton: The push button, or command button, is perhaps the most commonly used widget in any graphical user interface. Push (click) a button to command the computer to perform some action, or to answer a question. Typical buttons are OK, Apply, Cancel, Close, Yes, No and Help.
QRadioButton: A QRadioButton is an option button that can be switched on (checked) or off (unchecked). Radio buttons typically present the user with a "one of many" choice. In a group of radio buttons, only one radio button at a time can be checked; if the user selects another button, the previously selected button is switched off.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QLabel, QLineEdit, QPushButton, QRadioButton
class TemperatureConverterApp(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Temperature Converter")
self.setGeometry(100, 100, 300, 200) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create radio buttons for unit selection
self.celsius_radio = QRadioButton("Celsius")
self.fahrenheit_radio = QRadioButton("Fahrenheit")
# Create labels and input fields for temperature input and result
input_label = QLabel("Enter Temperature:")
self.input_field = QLineEdit()
result_label = QLabel("Result:")
self.result_field = QLineEdit()
self.result_field.setReadOnly(True)
# Create a convert button
convert_button = QPushButton("Convert")
convert_button.clicked.connect(self.convert_temperature)
# Create layout for unit selection
unit_layout = QVBoxLayout()
unit_layout.addWidget(self.celsius_radio)
unit_layout.addWidget(self.fahrenheit_radio)
# Create layout for temperature input and result
input_layout = QVBoxLayout()
input_layout.addWidget(input_label)
input_layout.addWidget(self.input_field)
input_layout.addWidget(result_label)
input_layout.addWidget(self.result_field)
input_layout.addWidget(convert_button)
# Create layout for central widget
layout = QVBoxLayout()
layout.addLayout(unit_layout)
layout.addLayout(input_layout)
# Set the layout for the central widget
central_widget.setLayout(layout)
# Set default unit to Celsius
self.celsius_radio.setChecked(True)
self.fahrenheit_radio.setChecked(False)
def convert_temperature(self):
try:
temperature = float(self.input_field.text())
if self.celsius_radio.isChecked():
# Convert from Celsius to Fahrenheit
converted_temperature = (temperature * 9/5) + 32
self.result_field.setText(f"{converted_temperature} °F")
else:
# Convert from Fahrenheit to Celsius
converted_temperature = (temperature - 32) * 5/9
self.result_field.setText(f"{converted_temperature} °C")
except ValueError:
self.result_field.setText("Invalid input")
def main():
app = QApplication(sys.argv)
window = TemperatureConverterApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a "QMainWindow" named "TemperatureConverterApp" with a central widget.
- Set the window's title and initial size.
- Create radio buttons (celsius_radio and fahrenheit_radio) for selecting the temperature unit (Celsius or Fahrenheit).
- Create labels and input fields for temperature input (input_field) and display the result (result_field).
- Create a "Convert" button and connect its click event to the "convert_temperature()" method.
- Create layouts to arrange widgets, including unit selection and temperature input/result.
- The "convert_temperature()" method converts the input temperature between Celsius and Fahrenheit based on the selected unit and updates the result field accordingly.
- In the main function, we create the PyQt application, create an instance of the "TemperatureConverterApp" class, show the window, and run the application's event loop.
Output:
Flowchart:
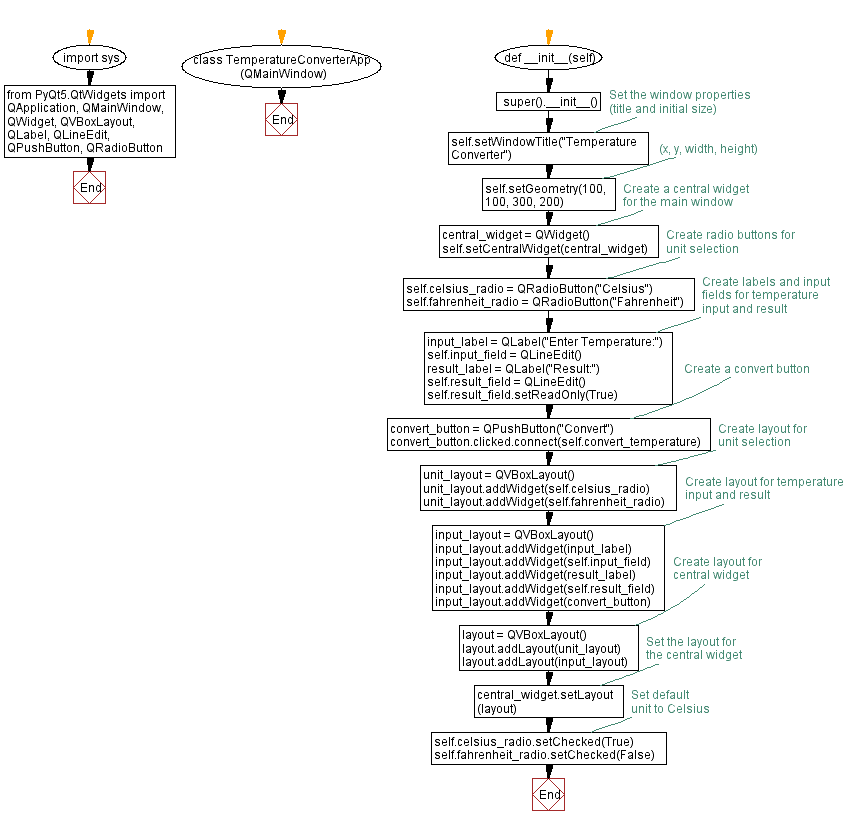
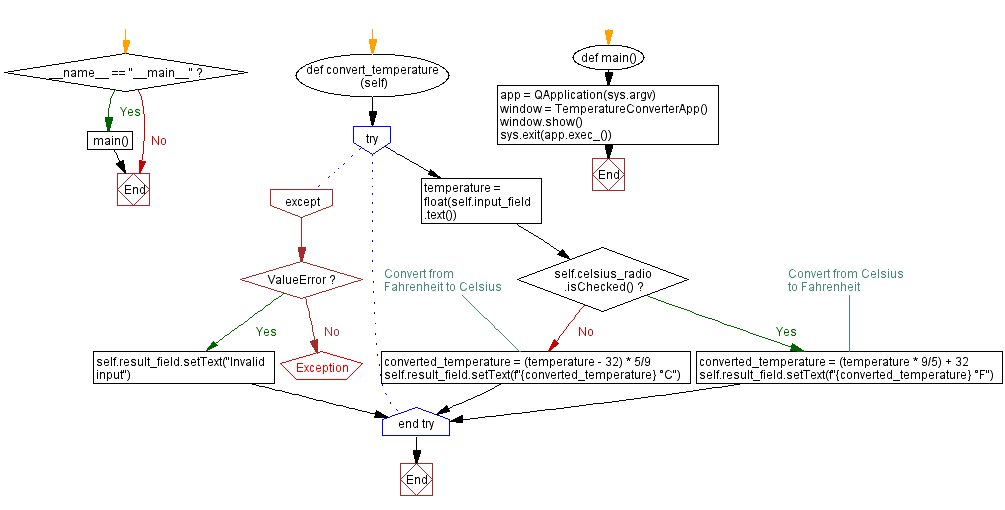
Go to:
Previous: Image viewer.
Next: User authentication.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.