Python PyQt program - Customize appearance
Write a Python program that customizes the appearance of a label text color, input field background color and a push button to close the dialog using PyQt.
From doc.qt.io:
QDialog Class: The QDialog class is the base class of dialog windows.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QLabel Class: The QLabel widget provides a text or image display.
QLineEdit Class: A line edit allows the user to enter and edit a single line of plain text with a useful collection of editing functions, including undo and redo, cut and paste, and drag and drop.
QPushButton: The push button, or command button, is perhaps the most commonly used widget in any graphical user interface. Push (click) a button to command the computer to perform some action, or to answer a question. Typical buttons are OK, Apply, Cancel, Close, Yes, No and Help.
QPalette Class: The QPalette class contains color groups for each widget state.
QColor Class: The QColor class provides colors based on RGB, HSV or CMYK values.
Sample Solution:
Python Code:
from PyQt5.QtWidgets import QDialog, QVBoxLayout, QLabel, QLineEdit, QPushButton
from PyQt5.QtGui import QPalette, QColor
class CustomDialog(QDialog):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Custom Dialog")
self.setGeometry(100, 100, 300, 150) # (x, y, width, height)
# Create a layout for the dialog
layout = QVBoxLayout()
# Create a QLabel with custom text color
label = QLabel("Input your name:")
palette = QPalette()
palette.setColor(QPalette.Foreground, QColor("red"))
label.setPalette(palette)
# Create a QLineEdit with custom background color
line_edit = QLineEdit()
line_edit.setStyleSheet("background-color: orange;")
# Create a QPushButton to close the dialog
close_button = QPushButton("Close")
close_button.clicked.connect(self.close)
# Add widgets to the layout
layout.addWidget(label)
layout.addWidget(line_edit)
layout.addWidget(close_button)
# Set the layout for the dialog
self.setLayout(layout)
def main():
dialog = CustomDialog()
dialog.exec_()
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a custom "QDialog" named "CustomDialog".
- Set the dialog's title and initial size.
- Create a QVBoxLayout to arrange widgets vertically.
- Create a QLabel with the text "Input your name:" and customize its text color to red using QPalette.
- Create a QLineEdit and customize its background color to light yellow using setStyleSheet.
- Create a QPushButton labeled "Close" and connect its click event to close the dialog.
- Add QLabel, QLineEdit, and QPushButton to the QVBoxLayout.
- Set the QVBoxLayout as the dialog layout.
- In the main() function, we create the PyQt application, create an instance of the "CustomDialog" class, show the window, and run the application's event loop.
Output:
Flowchart:
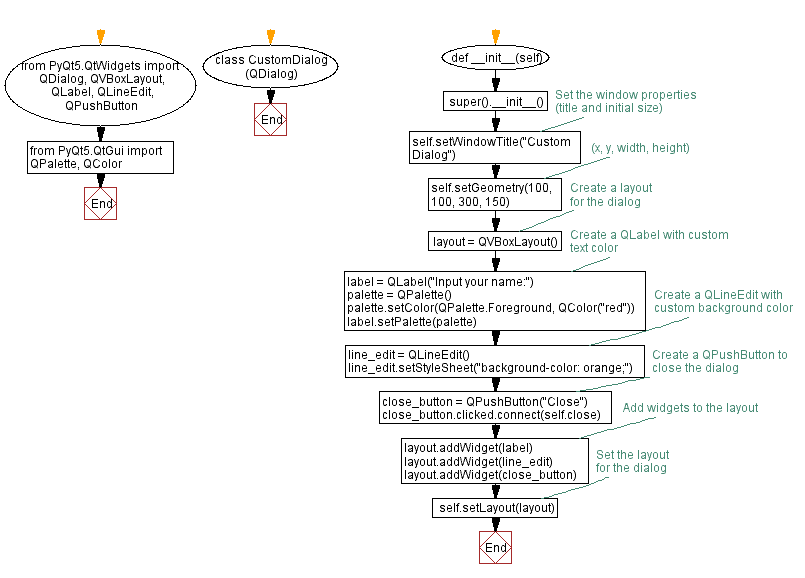
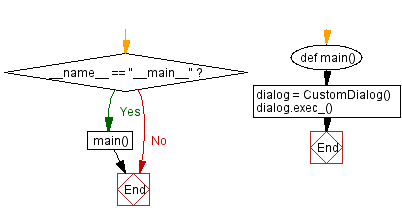
Python Code Editor:
Previous: Button color changer.
Next: Image viewer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics