Python PyQt program - Color picker
Python PyQt Widgets: Exercise-4 with Solution
Write a Python program to create a combobox with a list of colors using PyQt. When the user selects a color from the dropdown, change the main window background color.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QComboBox Class: The QComboBox widget is a combined button and popup list.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QWidget: The QWidget class is the base class of all user interface objects.
QColor Class: The QColor class provides colors based on RGB, HSV or CMYK values.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QTextEdit, QAction, QFileDialog, QVBoxLayout, QWidget
class TextEditorApp(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Text Editor")
self.setGeometry(100, 100, 600, 400) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create a QTextEdit widget for text input
self.text_edit = QTextEdit()
self.text_edit.setFontPointSize(14) # Set font size
# Create actions for Open and Save
open_action = QAction("Open", self)
save_action = QAction("Save", self)
# Connect actions to methods
open_action.triggered.connect(self.open_file)
save_action.triggered.connect(self.save_file)
# Create a menu bar with File menu
menu_bar = self.menuBar()
file_menu = menu_bar.addMenu("File")
file_menu.addAction(open_action)
file_menu.addAction(save_action)
# Create a layout for the central widget and add the QTextEdit
layout = QVBoxLayout()
layout.addWidget(self.text_edit)
# Set the layout for the central widget
central_widget.setLayout(layout)
def open_file(self):
options = QFileDialog.Options()
options |= QFileDialog.ReadOnly # Open file in read-only mode
file_path, _ = QFileDialog.getOpenFileName(self, "Open File", "", "Text Files (*.txt);;All Files (*)", options=options)
if file_path:
with open(file_path, 'r') as file:
self.text_edit.setPlainText(file.read())
def save_file(self):
options = QFileDialog.Options()
options |= QFileDialog.ReadOnly # Open file in read-only mode
file_path, _ = QFileDialog.getSaveFileName(self, "Save File", "", "Text Files (*.txt);;All Files (*)", options=options)
if file_path:
with open(file_path, 'w') as file:
file.write(self.text_edit.toPlainText())
def main():
app = QApplication(sys.argv)
window = TextEditorApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a 'QMainWindow' named ColorPickerApp with a central widget.
- Set the window's title and initial size.
- Make a 'QComboBox' widget ('color_combo') and populate it with the names of the colors.
- The "currentIndexChanged" signal of the combo box should be connected to the "change_color()" method, which will be called by the user when the color is selected.
- Based on the selected color from the combo box, "change_color()" sets the background color of the central widget.
- In the main() function, we create the PyQt application, create an instance of the "ColorPickerApp" class, show the window, and run the application's event loop.
Output:
Flowchart:
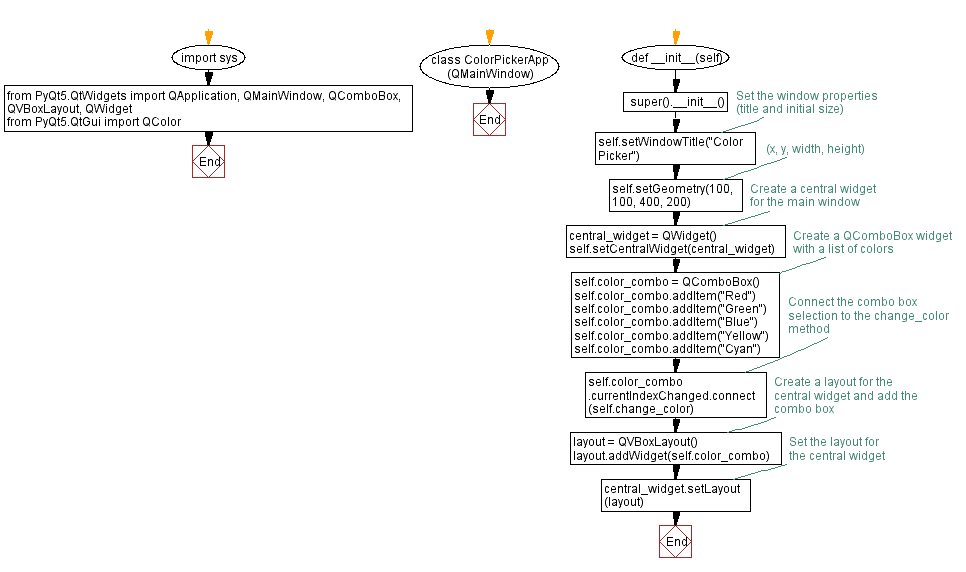
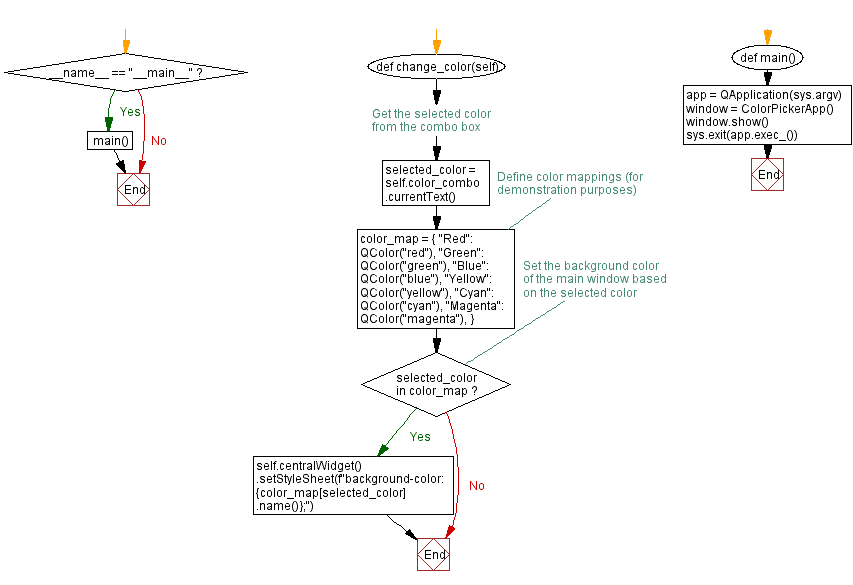
Python Code Editor:
Previous: Simple text editor.
Next: Button color changer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/pyqt/python-pyqt-widgets-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics